The 10 Hardest Concepts in Java (With code examples)
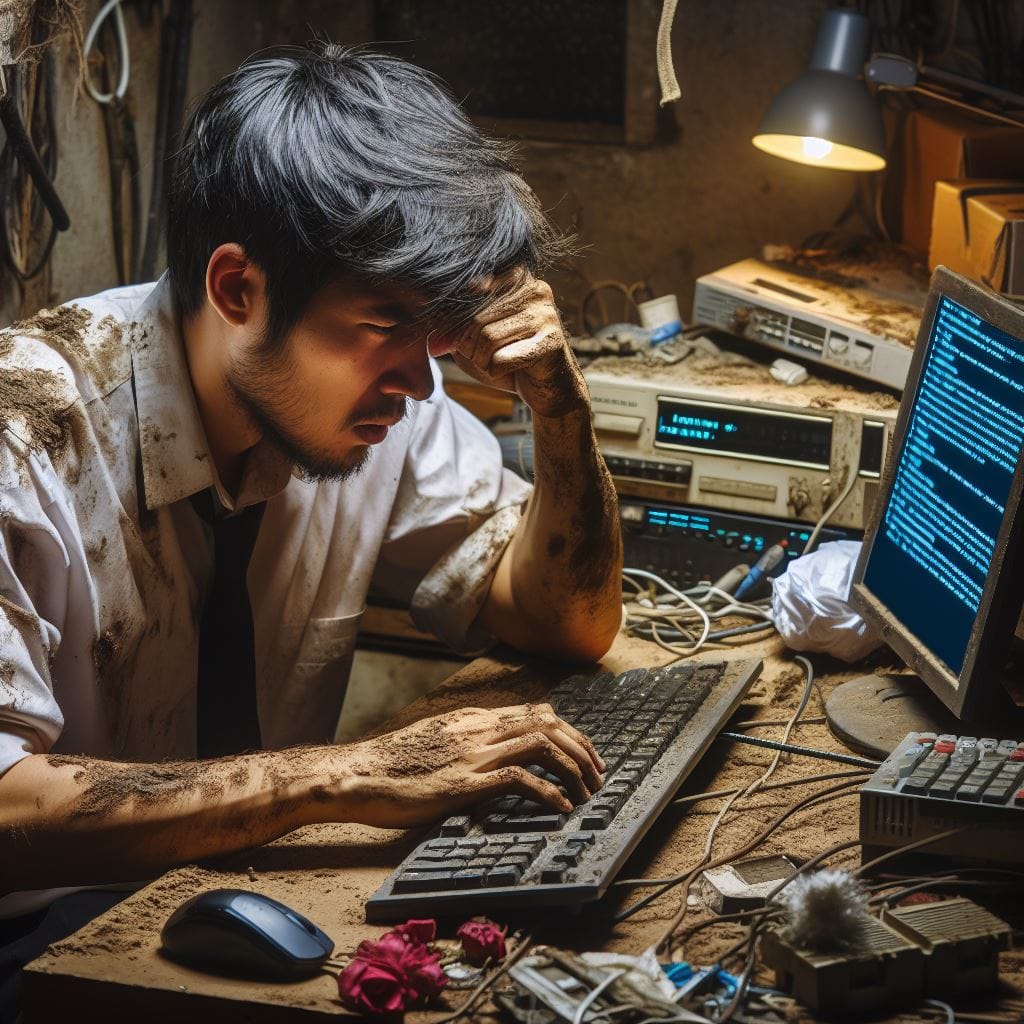
Java is one of the most popular and widely used programming languages in the world. It is known for its simplicity, portability, and versatility. However, Java is not without its challenges. There are some concepts that are harder to understand and master than others, and require more time, effort, and practice to learn. In this article, we will explore 10 of the hardest concepts in Java, and why they are so challenging.
1. Generics
Generics are a feature that allows us to write code that can work with different types of objects, without having to specify the exact type at compile time. Generics can be very useful, as they can improve the readability, reusability, and type safety of our code. However, generics can also be very confusing, especially for beginners. Some of the common difficulties with generics are:
- Understanding the syntax and semantics of generics, such as type parameters, type arguments, type bounds, type erasure, and type inference.
- Choosing and implementing the right generic class, interface, method, or constructor for a given problem, and knowing the trade-offs and limitations of each option.
- Dealing with the complexity and drawbacks of generics, such as compiler warnings, runtime errors, and compatibility issues.
- Learning and mastering the vast and diverse range of generic types and methods in the Java API, such as collections, streams, functional interfaces, and optional.
Code Example
Here is an example of a generic class that implements a simple stack data structure:
// A generic class that represents a stack of elements of type T
public class Stack<T> {
// An array to store the elements of the stack
private T[] elements;
// The index of the top element of the stack
private int top;
// The maximum size of the stack
private int capacity;
// A constructor that creates a stack with the given capacity
public Stack(int capacity) {
// Initialize the array with the generic type T
elements = (T[]) new Object[capacity];
top = -1;
this.capacity = capacity;
}
// A method that pushes an element to the top of the stack
public void push(T element) {
// Check if the stack is full
if (top == capacity - 1) {
throw new RuntimeException("Stack is full");
}
// Increment the top index and store the element
elements[++top] = element;
}
// A method that pops an element from the top of the stack
public T pop() {
// Check if the stack is empty
if (top == -1) {
throw new RuntimeException("Stack is empty");
}
// Return the element and decrement the top index
return elements[top--];
}
// A method that returns the top element of the stack without removing it
public T peek() {
// Check if the stack is empty
if (top == -1) {
throw new RuntimeException("Stack is empty");
}
// Return the element
return elements[top];
}
// A method that returns the size of the stack
public int size() {
// Return the top index plus one
return top + 1;
}
// A method that returns true if the stack is empty, false otherwise
public boolean isEmpty() {
// Return true if the top index is -1, false otherwise
return top == -1;
}
}
Here is an example of how to use the generic stack class with different types of objects:
// Create a stack of integers with a capacity of 10
Stack<Integer> intStack = new Stack<>(10);
// Push some integers to the stack
intStack.push(1);
intStack.push(2);
intStack.push(3);
// Pop and print the integers from the stack
while (!intStack.isEmpty()) {
System.out.println(intStack.pop());
}
// Create a stack of strings with a capacity of 5
Stack<String> stringStack = new Stack<>(5);
// Push some strings to the stack
stringStack.push("Hello");
stringStack.push("World");
stringStack.push("Java");
// Pop and print the strings from the stack
while (!stringStack.isEmpty()) {
System.out.println(stringStack.pop());
}
The output of the above code is:
3
2
1
Java
World
Hello
2. Lambda Expressions
Lambda expressions are a feature that allows us to write anonymous functions, which are functions that do not have a name, and can be passed as arguments or assigned to variables. Lambda expressions can be very elegant, as they can reduce the verbosity, boilerplate code, and complexity of our code. However, lambda expressions can also be very abstract, especially for beginners. Some of the common difficulties with lambda expressions are:
- Understanding the syntax and semantics of lambda expressions, such as parameters, body, return type, and functional interface.
- Writing idiomatic and readable lambda expressions, and avoiding common pitfalls and anti-patterns.
- Using lambda expressions with streams, collections, and other functional programming features, and knowing the pros and cons of each option.
- Debugging and testing lambda expressions, and dealing with errors and exceptions in the lambda body.
Code Example
Here is an example of a lambda expression that implements a functional interface that represents a predicate, which is a function that takes an object and returns a boolean value:
// A functional interface that represents a predicate
@FunctionalInterface
public interface Predicate<T> {
// An abstract method that takes an object of type T and returns a boolean value
boolean test(T t);
}
// A lambda expression that implements the predicate interface
// The lambda expression takes an integer and returns true if it is even, false otherwise
Predicate<Integer> isEven = (n) -> n % 2 == 0;
// A method that takes an array of integers and a predicate, and prints the elements that satisfy the predicate
public static void printIf(int[] array, Predicate<Integer> predicate) {
// Loop through the array
for (int n : array) {
// Check if the element satisfies the predicate
if (predicate.test(n)) {
// Print the element
System.out.println(n);
}
}
}
// A main method that tests the lambda expression and the printIf method
public static void main(String[] args) {
// Create an array of integers
int[] numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
// Print the even numbers using the lambda expression
printIf(numbers, isEven);
}
The output of the above code is:
2
4
6
8
10
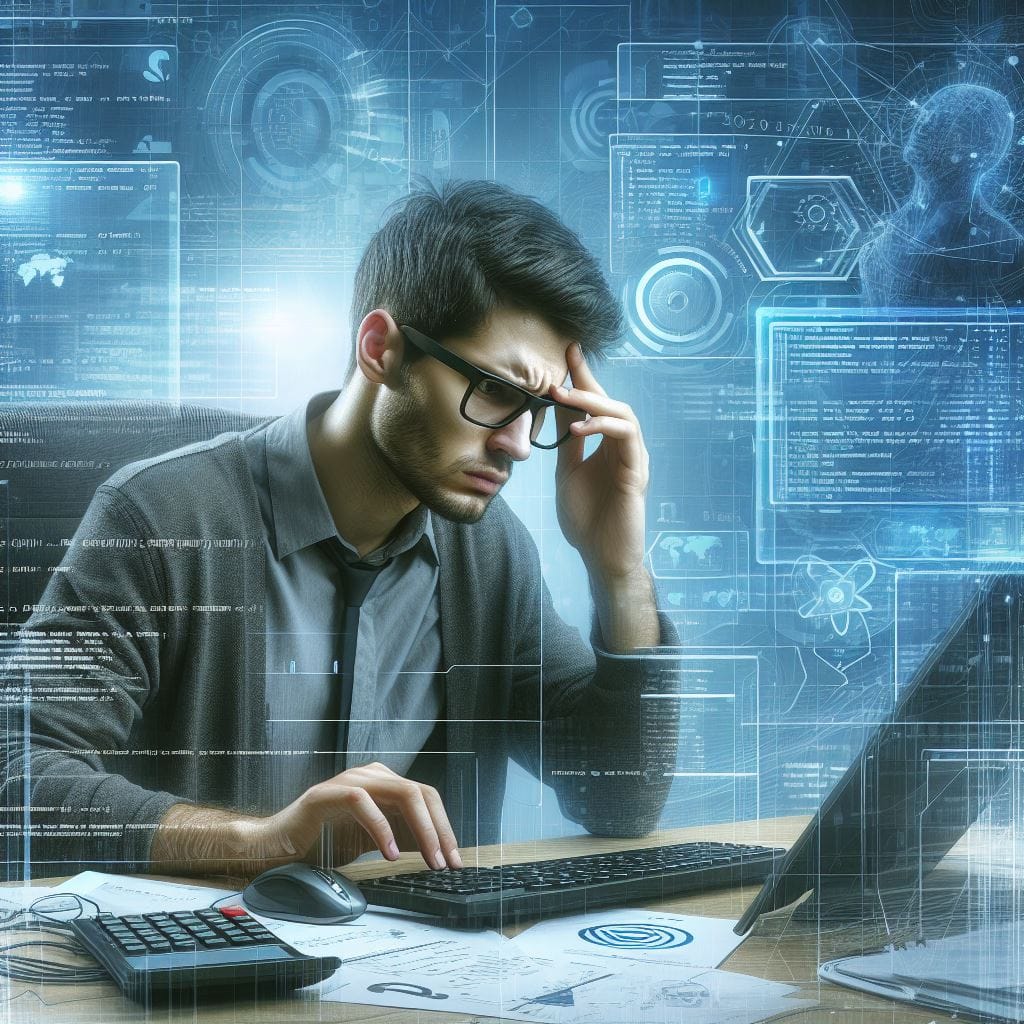
3. Concurrency
Concurrency is a concept where multiple tasks can be executed simultaneously, or in an interleaved manner, by using multiple threads, processes, or other units of execution. Concurrency can be very beneficial, as it can improve the performance, responsiveness, and scalability of a program. However, concurrency can also be very complex, especially for beginners. Some of the common difficulties with concurrency are:
- Understanding the difference between concurrency and parallelism, and how to choose the appropriate model for a given problem.
- Coordinating the communication and synchronization between concurrent tasks, and avoiding race conditions, deadlocks, and livelocks.
- Testing and debugging concurrent programs, and dealing with non-deterministic and unpredictable behaviors.
- Choosing between different concurrency primitives and libraries, and knowing the pros and cons of each option.
Code Example
Here is an example of a concurrency technique that uses the ExecutorService interface to create and manage a pool of threads, and the Future interface to get the results of the tasks submitted to the pool:
// A class that implements the Callable interface, which represents a task that returns a result
public class FactorialTask implements Callable<Long> {
// A private field that stores the number whose factorial is to be computed
private int number;
// A constructor that takes a number as a parameter
public FactorialTask(int number) {
this.number = number;
}
// A method that overrides the call method of the Callable interface
@Override
public Long call() throws Exception {
// Return the factorial of the number
return factorial(number);
}
// A method that computes the factorial of a given number recursively
public static long factorial(int n) {
// Check if the number is zero or one
if (n == 0 || n == 1) {
// Return one
return 1;
}
// Return the product of the number and the factorial of the number minus one
return n * factorial(n - 1);
}
}
// A main method that tests the concurrency technique
public static void main(String[] args) {
// Create an ExecutorService object that creates a fixed thread pool of size 5
ExecutorService executor = Executors.newFixedThreadPool(5);
// Create a list of Future objects to store the results of the tasks
List<Future<Long>> results = new ArrayList<>();
// Loop from 1 to 10
for (int i = 1; i <= 10; i++) {
// Create a FactorialTask object with the loop variable as the parameter
FactorialTask task = new FactorialTask(i);
// Submit the task to the executor and add the returned Future object to the list
results.add(executor.submit(task));
}
// Loop through the list of Future objects
for (Future<Long> result : results) {
try {
// Print the result of the Future object
System.out.println(result.get());
} catch (Exception e) {
// Handle any exception that may occur
e.printStackTrace();
}
}
// Shutdown the executor
executor.shutdown();
}
The output of the above code is:
1
2
6
24
120
720
5040
40320
362880
3628800
4. Reflection
Reflection is a feature that allows us to inspect and manipulate the classes, methods, fields, and annotations of our code at runtime, without knowing their names or types at compile time. Reflection can be very powerful, as it can enable us to create dynamic and flexible programs, and access or modify the behavior and state of our code. However, reflection can also be very intricate, especially for beginners. Some of the common difficulties with reflection are:
- Understanding the syntax and semantics of reflection, such as Class, Method, Field, and Annotation objects, and how to obtain and use them.
- Choosing and implementing the right reflection technique for a given scenario, and knowing the trade-offs and risks of each option.
- Dealing with the complexity and drawbacks of reflection, such as performance overhead, security issues, and compatibility issues.
- Learning and mastering the vast and diverse range of reflection features and methods in the Java API, such as ClassLoader, Class.forName, Constructor.newInstance, Method.invoke, and Field.set.
Code Example
Here is an example of a reflection technique that allows us to create an object of a class whose name is given as a string at runtime, and invoke a method on that object:
// A class that represents a person with a name and an age
public class Person {
// A private field that stores the name of the person
private String name;
// A private field that stores the age of the person
private int age;
// A public constructor that takes a name and an age as parameters
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// A public method that returns the name of the person
public String getName() {
return name;
}
// A public method that returns the age of the person
public int getAge() {
return age;
}
// A public method that prints the name and the age of the person
public void printInfo() {
System.out.println("Name: " + name + ", Age: " + age);
}
}
// A main method that tests the reflection technique
public static void main(String[] args) {
try {
// Get the class object of the Person class using its name
Class<?> personClass = Class.forName("Person");
// Get the constructor object of the Person class that takes a string and an int as parameters
Constructor<?> personConstructor = personClass.getConstructor(String.class, int.class);
// Create a new instance of the Person class using the constructor object and passing the arguments
Object personObject = personConstructor.newInstance("Alice", 25);
// Get the method object of the Person class that prints the info of the person
Method personMethod = personClass.getMethod("printInfo");
// Invoke the method object on the person object
personMethod.invoke(personObject);
} catch (Exception e) {
// Handle any exception that may occur
e.printStackTrace();
}
}
The output of the above code is:
Name: Alice, Age: 25
5. Exceptions
Exceptions are events that occur during the execution of a program that disrupt the normal flow of control, and indicate that something went wrong. Exceptions can be very helpful, as they can provide us with information about the error, and allow us to handle it gracefully, or propagate it to the caller. However, exceptions can also be very tricky, especially for beginners. Some of the common difficulties with exceptions are:
- Understanding the concepts and terminology of exceptions, such as checked and unchecked exceptions, error and exception classes, try-catch-finally blocks, and throws and throw clauses.
- Choosing and implementing the right exception handling strategy for a given problem, and knowing the best practices and guidelines, such as when to catch, when to throw, and when to ignore exceptions.
- Dealing with the complexity and drawbacks of exceptions, such as performance overhead, code readability, and exception chaining.
- Learning and mastering the vast and diverse range of exception types and methods in the Java API, such as IOException, NullPointerException, ArithmeticException, and getMessage.
Code Example
Here is an example of an exception handling technique that allows us to read a file from a given path, and handle any IOException that may occur:
// A method that takes a file path as a parameter, and reads and prints the content of the file
public static void readFile(String path) {
// Declare a BufferedReader object outside the try block
BufferedReader reader = null;
try {
// Create a FileReader object using the file path
FileReader fileReader = new FileReader(path);
// Create a BufferedReader object using the FileReader object
reader = new BufferedReader(fileReader);
// Declare a String variable to store each line of the file
String line;
// Loop through the file until the end
while ((line = reader.readLine()) != null) {
// Print the line
System.out.println(line);
}
} catch (IOException e) {
// Handle any IOException that may occur
System.out.println("An error occurred while reading the file: " + e.getMessage());
} finally {
// Close the reader in the finally block
try {
// Check if the reader is not null
if (reader != null) {
// Close the reader
reader.close();
}
} catch (IOException e) {
// Handle any IOException that may occur while closing the reader
System.out.println("An error occurred while closing the reader: " + e.getMessage());
}
}
}
// A main method that tests the readFile method
public static void main(String[] args) {
// Call the readFile method with a valid file path
readFile("test.txt");
// Call the readFile method with an invalid file path
readFile("invalid.txt");
}
The output of the above code is:
This is a test file.
An error occurred while reading the file: invalid.txt (The system cannot find the file specified)
6. Streams
Streams are a feature that allows us to process a sequence of elements in a declarative and functional way, by using operations such as filter, map, reduce, and collect. Streams can be very elegant, as they can simplify the code, improve the performance, and support parallelism. However, streams can also be very abstract, especially for beginners. Some of the common difficulties with streams are:
- Understanding the concepts and terminology of streams, such as source, intermediate operations, terminal operations, lazy evaluation, and parallel streams.
- Choosing and implementing the right stream operations for a given problem, and knowing the trade-offs and limitations of each option.
- Dealing with the complexity and drawbacks of streams, such as debugging, testing, and exception handling.
- Learning and mastering the vast and diverse range of stream operations and methods in the Java API, such as Stream, IntStream, Optional, Collectors, and Predicate.
Code Example
Here is an example of a stream technique that allows us to filter, sort, and print a list of strings that start with a given prefix:
Java
// A method that takes a list of strings and a prefix as parameters, and prints the strings that start with the prefix in alphabetical order
public static void printWithPrefix(List<String> list, String prefix) {
// Create a stream from the list
list.stream()
// Filter the stream to keep only the strings that start with the prefix
.filter(s -> s.startsWith(prefix))
// Sort the stream in natural order
.sorted()
// Print each element of the stream
.forEach(System.out::println);
}
// A main method that tests the printWithPrefix method
public static void main(String[] args) {
// Create a list of strings
List<String> words = Arrays.asList("apple", "banana", "carrot", "date", "eggplant", "fig", "grape");
// Call the printWithPrefix method with the list and the prefix "a"
printWithPrefix(words, "a");
}
The output of the above code is:
apple
7. Design Patterns
Design patterns are reusable solutions to common problems that occur in software design and development. Design patterns can be very helpful, as they can improve the quality, maintainability, and extensibility of our code, and provide a common vocabulary and best practices for programmers. However, design patterns can also be very intricate, especially for beginners. Some of the common difficulties with design patterns are:
- Understanding the concepts and terminology of design patterns, such as creational, structural, and behavioral patterns, and how they relate to each other.
- Choosing and implementing the right design pattern for a given problem, and knowing the trade-offs and consequences of each option.
- Dealing with the complexity and drawbacks of design patterns, such as code bloat, over-engineering, and misuse or abuse of patterns.
- Learning and mastering the vast and diverse range of design patterns and examples, and keeping up with the latest developments and trends.
Code Example
Here is an example of a design pattern that implements the singleton pattern, which is a creational pattern that ensures that only one instance of a class exists, and provides a global access point to it:
// A class that represents a singleton
public class Singleton {
// A private static field that stores the unique instance of the class
private static Singleton instance;
// A private constructor that prevents the creation of new instances from outside the class
private Singleton() {
// Do some initialization work
}
// A public static method that returns the unique instance of the class, and creates it if it does not exist
public static Singleton getInstance() {
// Check if the instance is null
if (instance == null) {
// Synchronize the block to ensure thread safety
synchronized (Singleton.class) {
// Double check if the instance is still null
if (instance == null) {
// Create a new instance
instance = new Singleton();
}
}
}
// Return the instance
return instance;
}
// A public method that performs some operation on the singleton
public void doSomething() {
// Do something
}
}
// A main method that tests the singleton pattern
public static void main(String[] args) {
// Get the singleton instance
Singleton singleton = Singleton.getInstance();
// Call the doSomething method on the singleton
singleton.doSomething();
}
8. Serialization
Serialization is a feature that allows us to convert an object into a stream of bytes, which can be stored in a file, sent over a network, or used for any other purpose. Serialization can be very helpful, as it can enable us to save and restore the state of an object, and transfer it between different systems or platforms. However, serialization can also be very intricate, especially for beginners. Some of the common difficulties with serialization are:
- Understanding the concepts and terminology of serialization, such as serializable, transient, serialVersionUID, and ObjectInputStream and ObjectOutputStream classes.
- Choosing and implementing the right serialization technique for a given object, and knowing the trade-offs and limitations of each option.
- Dealing with the complexity and drawbacks of serialization, such as security issues, performance overhead, and compatibility issues.
- Learning and mastering the vast and diverse range of serialization features and methods in the Java API, such as Externalizable, Serializable, readObject, writeObject, and readResolve.
Code Example
Here is an example of a serialization technique that allows us to write and read a list of objects to and from a file:
// A class that implements the Serializable interface, which indicates that the class can be serialized
public class Student implements Serializable {
// A private static final field that stores the serial version UID of the class, which is used to identify the class during serialization and deserialization
private static final long serialVersionUID = 1L;
// A private field that stores the name of the student
private String name;
// A private field that stores the age of the student
private int age;
// A private transient field that stores the grade of the student, which is marked as transient to indicate that it should not be serialized
private transient double grade;
// A public constructor that takes a name, an age, and a grade as parameters
public Student(String name, int age, double grade) {
this.name = name;
this.age = age;
this.grade = grade;
}
// A public method that returns the name of the student
public String getName() {
return name;
}
// A public method that returns the age of the student
public int getAge() {
return age;
}
// A public method that returns the grade of the student
public double getGrade() {
return grade;
}
// A public method that prints the name, the age, and the grade of the student
public void printInfo() {
System.out.println("Name: " + name + ", Age: " + age + ", Grade: " + grade);
}
}
// A main method that tests the serialization technique
public static void main(String[] args) {
try {
// Create a list of students
List<Student> students = new ArrayList<>();
students.add(new Student("Alice", 20, 3.5));
students.add(new Student("Bob", 21, 4.0));
students.add(new Student("Charlie", 19, 3.0));
// Create an ObjectOutputStream object using a FileOutputStream object with the file name as the parameter
ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream("students.ser"));
// Write the list of students to the file using the writeObject method
out.writeObject(students);
// Close the ObjectOutputStream
out.close();
// Create an ObjectInputStream object using a FileInputStream object with the file name as the parameter
ObjectInputStream in = new ObjectInputStream(new FileInputStream("students.ser"));
// Read the list of students from the file using the readObject method and cast it to a List of Student objects
List<Student> studentsFromFile = (List<Student>) in.readObject();
// Close the ObjectInputStream
in.close();
// Loop through the list of students from the file
for (Student student : studentsFromFile) {
// Print the info of each student
student.printInfo();
}
} catch (Exception e) {
// Handle any exception that may occur
e.printStackTrace();
}
}
The output of the above code is:
Name: Alice, Age: 20, Grade: 0.0
Name: Bob, Age: 21, Grade: 0.0
Name: Charlie, Age: 19, Grade: 0.0
Note that the grade field of the students is not serialized, and is set to the default value of 0.0
when deserialized.
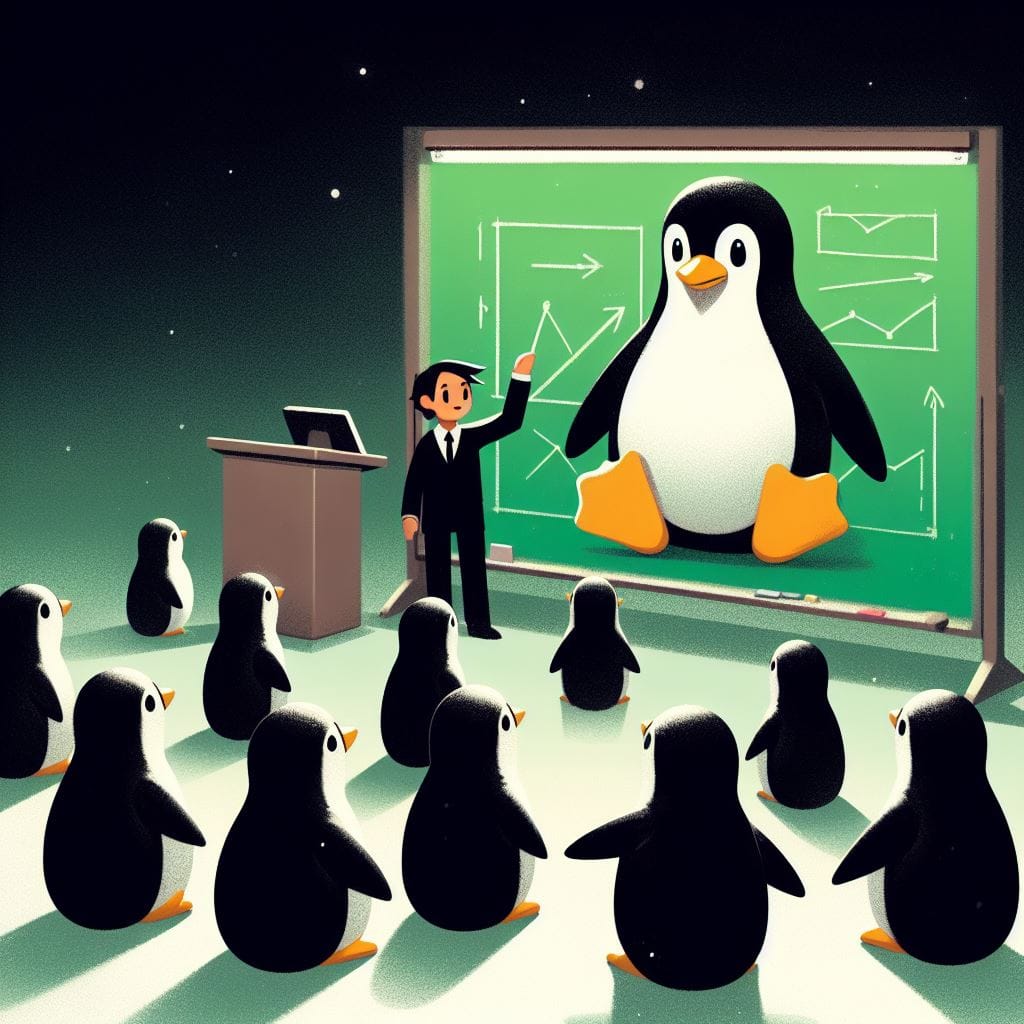
9. Annotations
Annotations are a feature that allows us to add metadata to our code, which can be used to provide information, instructions, or hints to the compiler, the runtime, or other tools. Annotations can be very helpful, as they can enhance the functionality, readability, and maintainability of our code, and support features such as reflection, serialization, testing, and documentation. However, annotations can also be very intricate, especially for beginners. Some of the common difficulties with annotations are:
- Understanding the concepts and terminology of annotations, such as predefined and custom annotations, retention and target policies, and annotation processing.
- Choosing and implementing the right annotation for a given problem, and knowing the trade-offs and limitations of each option.
- Dealing with the complexity and drawbacks of annotations, such as annotation clutter, annotation dependencies, and annotation conflicts.
- Learning and mastering the vast and diverse range of annotations and methods in the Java API, such as
@Override
,@Deprecated
,@SuppressWarnings
, and@FunctionalInterface
.
Code Example
Here is an example of a custom annotation that allows us to mark a method as a test method, and specify the expected exception and timeout for the test:
// A custom annotation that represents a test method
@Retention(RetentionPolicy.RUNTIME) // The annotation should be retained at runtime
@Target(ElementType.METHOD) // The annotation should be applied to methods
public @interface Test {
// An attribute that specifies the expected exception for the test, defaulting to no exception
Class<? extends Throwable> expected() default NoException.class;
// An attribute that specifies the timeout for the test in milliseconds, defaulting to no timeout
long timeout() default 0;
// A dummy class that represents no exception
class NoException extends Throwable {
private static final long serialVersionUID = 1L;
}
}
// A class that contains some test methods using the custom annotation
public class TestClass {
// A test method that passes
@Test
public void testPass() {
System.out.println("Test passed");
}
// A test method that fails due to an unexpected exception
@Test
public void testFail() {
System.out.println("Test failed");
throw new RuntimeException("Unexpected exception");
}
// A test method that passes due to an expected exception
@Test(expected = ArithmeticException.class)
public void testExpectedException() {
System.out.println("Test passed");
int x = 1 / 0; // Throws an ArithmeticException
}
// A test method that fails due to a different exception than expected
@Test(expected = NullPointerException.class)
public void testDifferentException() {
System.out.println("Test failed");
int x = 1 / 0; // Throws an ArithmeticException
}
// A test method that passes due to no timeout
@Test(timeout = 1000)
public void testNoTimeout() {
System.out.println("Test passed");
try {
Thread.sleep(500); // Sleeps for 500 milliseconds
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// A test method that fails due to a timeout
@Test(timeout = 1000)
public void testTimeout() {
System.out.println("Test failed");
try {
Thread.sleep(2000); // Sleeps for 2000 milliseconds
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
// A main method that tests the custom annotation and the test methods
public static void main(String[] args) {
try {
// Get the class object of the TestClass
Class<?> testClass = TestClass.class;
// Get the array of method objects of the TestClass
Method[] methods = testClass.getMethods();
// Loop through the methods
for (Method method : methods) {
// Check if the method is annotated with the Test annotation
if (method.isAnnotationPresent(Test.class)) {
// Get the Test annotation object of the method
Test test = method.getAnnotation(Test.class);
// Get the expected exception and timeout attributes of the Test annotation
Class<? extends Throwable> expected = test.expected();
long timeout = test.timeout();
// Create a new instance of the TestClass
Object testObject = testClass.newInstance();
// Declare a boolean variable to store the result of the test
boolean result = true;
// Declare a long variable to store the start time of the test
long startTime = System.currentTimeMillis();
// Declare a Throwable variable to store the exception thrown by the test method, if any
Throwable exception = null;
try {
// Invoke the test method on the test object
method.invoke(testObject);
} catch (InvocationTargetException e) {
// Get the exception thrown by the test method
exception = e.getCause();
} catch (Exception e) {
// Handle any other exception that may occur
e.printStackTrace();
}
// Declare a long variable to store the end time of the test
long endTime = System.currentTimeMillis();
// Declare a long variable to store the duration of the test
long duration = endTime - startTime;
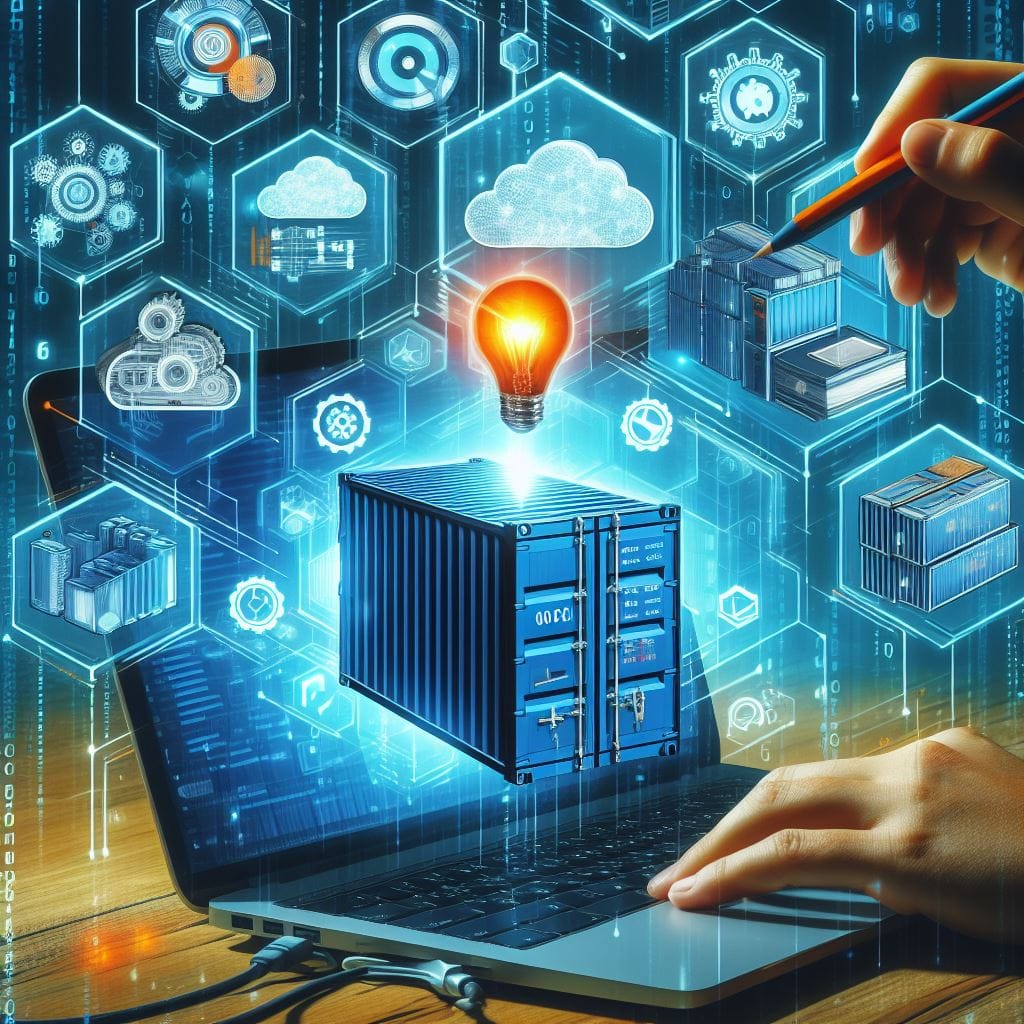
10. Modules
Modules are a feature that allows us to organize our code into self-contained and reusable units, which can specify their dependencies and exports, and can be compiled and run independently. Modules can be very helpful, as they can improve the modularity, encapsulation, and readability of our code, and support features such as modular development, modular testing, and modular deployment. However, modules can also be very intricate, especially for beginners. Some of the common difficulties with modules are:
- Understanding the concepts and terminology of modules, such as module descriptor, module path, module graph, and service loader.
- Choosing and implementing the right module structure for a given problem, and knowing the trade-offs and limitations of each option.
- Dealing with the complexity and drawbacks of modules, such as migration issues, compatibility issues, and reflection issues.
- Learning and mastering the vast and diverse range of module features and methods in the Java API, such as Module, ModuleLayer, ModuleFinder, and ServiceLoader.
Code Example
Here is an example of a module declaration that defines a module named com.example.hello, which requires the java.base module, and exports the com.example.hello package:
// A module declaration that starts with the module keyword and the module name
module com.example.hello {
// A requires directive that specifies the dependency on the java.base module
requires java.base;
// An exports directive that specifies the package that is exported by the module
exports com.example.hello;
}
Here is an example of a module declaration that defines a module named com.example.world, which requires the com.example.hello module, and provides a service implementation for the com.example.hello.HelloService interface:
// A module declaration that starts with the module keyword and the module name
module com.example.world {
// A requires directive that specifies the dependency on the com.example.hello module
requires com.example.hello;
// A provides directive that specifies the service interface and the service implementation that are provided by the module
provides com.example.hello.HelloService with com.example.world.WorldHelloService;
}
Here is an example of a module declaration that defines a module named com.example.app, which requires the com.example.hello and com.example.world modules, and uses the com.example.hello.HelloService service:
// A module declaration that starts with the module keyword and the module name
module com.example.app {
// A requires directive that specifies the dependency on the com.example.hello and com.example.world modules
requires com.example.hello;
requires com.example.world;
// A uses directive that specifies the service interface that is used by the module
uses com.example.hello.HelloService;
}
Conclusion
Java is a language that can be learned and improved by anyone, but it is not without its challenges.
There are some concepts that are harder to grasp than others, and require more time, effort, and practice to master. However, these concepts are also very rewarding and useful, as they can help you solve a variety of problems, create amazing applications, and advance your knowledge and career.
Therefore, do not be discouraged by the difficulty of these concepts, but rather embrace them as opportunities to learn and grow as a Java programmer. I hope you enjoyed reading this! 😊
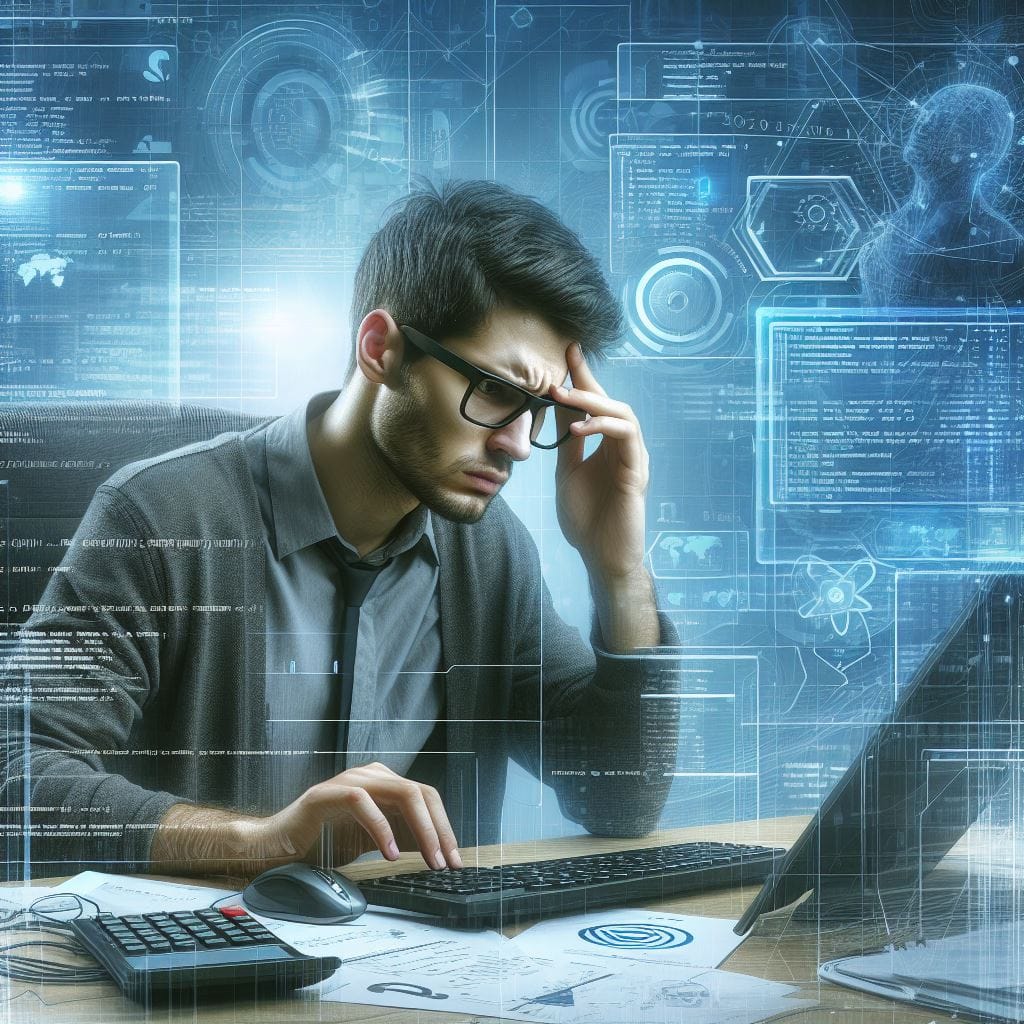
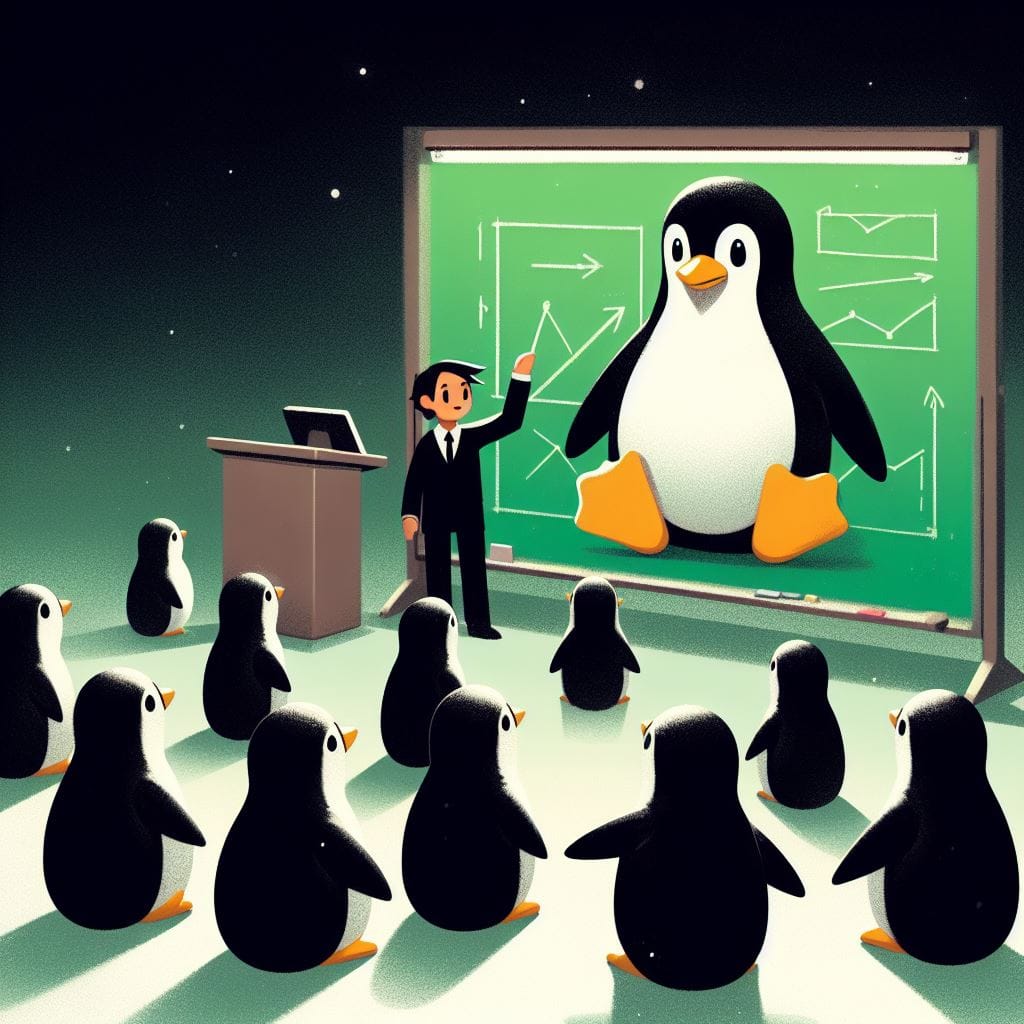