The 10 Most Difficult Programming Concepts to Grasp
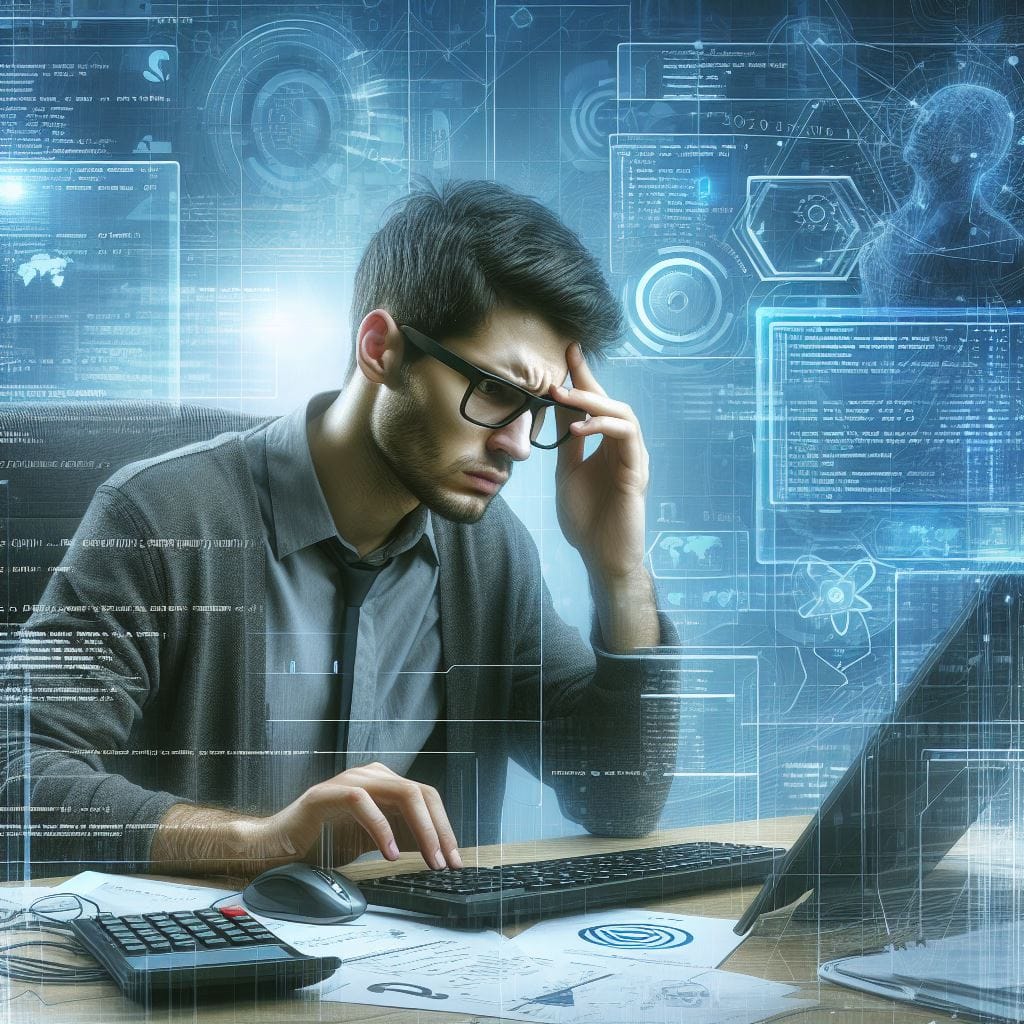
Programming is a skill that requires a lot of logical thinking, creativity, and patience. It can also be very rewarding and fun, especially when you can create something useful or beautiful with code. However, programming is not always easy. There are some concepts that are notoriously difficult to understand, even for experienced programmers. In this article, we will explore 10 of the most difficult programming concepts to grasp, and why they are so challenging.
1. Recursion
Recursion is a technique where a function calls itself repeatedly until a base case is reached. Recursion can be very useful for solving problems that involve breaking down a complex task into smaller and simpler subtasks, such as traversing a tree, sorting an array, or generating a fractal. However, recursion can also be very confusing, especially for beginners. Some of the common difficulties with recursion are:
- Understanding the base case and the recursive case, and how they relate to each other.
- Keeping track of the call stack and the return values of each recursive call.
- Avoiding infinite recursion and stack overflow errors.
- Choosing between recursion and iteration, and knowing the trade-offs between them.
2. Pointers
Pointers are variables that store the memory address of another variable, rather than its value. Pointers can be very powerful, as they allow us to manipulate data indirectly, pass data by reference, create dynamic data structures, and implement low-level operations. However, pointers can also be very tricky, especially for beginners. Some of the common difficulties with pointers are:
- Understanding the difference between pointers and values, and how to dereference and reference them correctly.
- Managing memory allocation and deallocation, and avoiding memory leaks and dangling pointers.
- Using pointers with arrays, strings, structs, and functions, and knowing the syntax and semantics of each case.
- Debugging pointer-related errors, such as segmentation faults and invalid pointer operations.
3. Concurrency
Concurrency is a concept where multiple tasks can be executed simultaneously, or in an interleaved manner, by using multiple threads, processes, or other units of execution. Concurrency can be very beneficial, as it can improve the performance, responsiveness, and scalability of a program. However, concurrency can also be very complex, especially for beginners. Some of the common difficulties with concurrency are:
- Understanding the difference between concurrency and parallelism, and how to choose the appropriate model for a given problem.
- Coordinating the communication and synchronization between concurrent tasks, and avoiding race conditions, deadlocks, and livelocks.
- Testing and debugging concurrent programs, and dealing with non-deterministic and unpredictable behaviors.
- Choosing between different concurrency primitives and libraries, and knowing the pros and cons of each option.
4. Functional Programming
Functional programming is a paradigm where programs are composed of pure functions, which are functions that do not have any side effects, and do not depend on or modify any external state. Functional programming can be very elegant, as it can reduce the complexity, bugs, and boilerplate code of a program. However, functional programming can also be very abstract, especially for beginners. Some of the common difficulties with functional programming are:
- Understanding the concepts and terminology of functional programming, such as higher-order functions, closures, currying, partial application, lazy evaluation, and monads.
- Adopting a functional mindset, and thinking in terms of functions, rather than variables, loops, and statements.
- Writing idiomatic and readable functional code, and avoiding common pitfalls and anti-patterns.
- Integrating functional programming with other paradigms and languages, and knowing when and how to use it.
5. Object-Oriented Programming
Object-oriented programming is a paradigm where programs are composed of objects, which are entities that have attributes and behaviors, and can interact with each other through messages. Object-oriented programming can be very intuitive, as it can model the real world and its entities in a natural way. However, object-oriented programming can also be very intricate, especially for beginners. Some of the common difficulties with object-oriented programming are:
- Understanding the concepts and principles of object-oriented programming, such as classes, objects, inheritance, polymorphism, abstraction, and encapsulation.
- Designing and implementing a good object-oriented system, and following the best practices and design patterns.
- Dealing with the complexity and drawbacks of object-oriented programming, such as code bloat, tight coupling, and inheritance hierarchies.
- Balancing between object-oriented programming and other paradigms and languages, and knowing when and how to use it.
6. Algorithms and Data Structures
Algorithms and data structures are the core of computer science and programming. Algorithms are the steps or rules that define how to solve a problem or perform a task, and data structures are the ways of organizing and storing data in a computer. Algorithms and data structures can be very fascinating, as they can reveal the beauty and logic of computation and mathematics. However, algorithms and data structures can also be very challenging, especially for beginners. Some of the common difficulties with algorithms and data structures are:
- Understanding the concepts and terminology of algorithms and data structures, such as complexity, efficiency, correctness, and optimality.
- Choosing and implementing the right algorithm and data structure for a given problem, and knowing the trade-offs and limitations of each option.
- Analyzing and comparing the performance and suitability of different algorithms and data structures, and using the appropriate tools and techniques, such as Big O notation, recurrence relations, and empirical testing.
- Learning and mastering the vast and diverse range of algorithms and data structures, and keeping up with the latest developments and trends.
7. Cryptography
Cryptography is the science and art of securing and protecting information and communication, by using techniques such as encryption, decryption, hashing, digital signatures, and authentication. Cryptography can be very important, as it can ensure the confidentiality, integrity, and authenticity of data and messages, and prevent unauthorized access and tampering. However, cryptography can also be very intricate, especially for beginners. Some of the common difficulties with cryptography are:
- Understanding the concepts and terminology of cryptography, such as keys, ciphers, modes, algorithms, and protocols.
- Choosing and implementing the right cryptographic technique for a given scenario, and knowing the trade-offs and risks of each option.
- Evaluating and verifying the security and robustness of different cryptographic techniques, and using the appropriate tools and methods, such as cryptanalysis, proofs, and attacks.
- Following the best practices and standards of cryptography, and avoiding common pitfalls and mistakes.
8. Machine Learning
Machine learning is a branch of artificial intelligence that deals with creating and using systems that can learn from data and experience, and perform tasks that are difficult or impossible to program explicitly, such as recognition, prediction, classification, and recommendation. Machine learning can be very exciting, as it can enable new and innovative applications and solutions, and unlock the potential of data and intelligence. However, machine learning can also be very complex, especially for beginners. Some of the common difficulties with machine learning are:
- Understanding the concepts and terminology of machine learning, such as models, features, labels, training, testing, validation, and evaluation.
- Choosing and implementing the right machine learning technique for a given problem, and knowing the trade-offs and assumptions of each option.
- Tuning and optimizing the performance and accuracy of different machine learning techniques, and using the appropriate tools and techniques, such as hyperparameters, regularization, cross-validation, and metrics.
- Dealing with the challenges and limitations of machine learning, such as data quality, scalability, interpretability, and ethics.
9. Compilers
Compilers are programs that translate source code written in a high-level programming language, such as C, Java, or Python, into executable code written in a low-level language, such as assembly or machine code. Compilers can be very essential, as they can enable programmers to write code in a more expressive, abstract, and portable way, and run it on different platforms and devices. However, compilers can also be very intricate, especially for beginners. Some of the common difficulties with compilers are:
- Understanding the concepts and terminology of distributed systems, such as nodes, processes, messages, protocols, and consensus.
- Designing and implementing a distributed system for a given problem, and following the best practices and techniques, such as fault tolerance, replication, consistency, and load balancing.
- Testing and debugging a distributed system, and dealing with failures, delays, and inconsistencies in the network and the components.
- Choosing between different distributed systems models and architectures, and knowing the pros and cons of each option.
Conclusion
Programming is a skill that can be learned and improved by anyone, but it is not without its challenges. There are some concepts that are more difficult to grasp than others, and require more time, effort, and practice to master.
However, these concepts are also very rewarding and useful, as they can help you solve a variety of problems, create amazing applications, and advance your knowledge and career.
Therefore, do not be discouraged by the difficulty of these concepts, but rather embrace them as opportunities to learn and grow as a programmer.