20 Key Computer Science Concepts Every Developer Should Know
Computer Science is a vast and diverse field that encompasses many sub-disciplines and applications. However, there are some fundamental concepts that are common to most areas of Computer Science and are essential for any developer to master.
In this blog post, I will introduce 20 of these key concepts, along with some examples and explanations. Note that this is not an exhaustive list, nor a definitive one. There may be other concepts that are equally or more important, depending on the context and the problem domain. This is just my personal selection, based on my own experience and perspective.
1. Algorithms
An algorithm is a step-by-step procedure for solving a problem or performing a task. Algorithms are the core of Computer Science, as they provide the logic and instructions for computers to execute. Algorithms can be expressed in various ways, such as natural language, pseudocode, flowcharts, or programming languages. Algorithms can be classified by their complexity, efficiency, correctness, optimality, and other criteria. Some examples of well-known algorithms are:
- Binary search: an algorithm for finding an element in a sorted array, by repeatedly dividing the array into two halves and comparing the middle element with the target value.
- Merge sort: an algorithm for sorting an array, by recursively dividing the array into smaller subarrays, sorting them, and merging them back together.
- Dijkstra’s algorithm: an algorithm for finding the shortest path between two nodes in a weighted graph, by maintaining a set of nodes with the minimum distance from the source node, and updating their distances and predecessors as new nodes are explored.
- RSA: an algorithm for public-key cryptography, by using large prime numbers and modular arithmetic to generate public and private keys, and encrypt and decrypt messages.
2. Data Structures
A data structure is a way of organizing and storing data in a computer, such that it can be accessed and manipulated efficiently and effectively. Data structures are closely related to algorithms, as different data structures may suit different algorithms better, and vice versa. Data structures can be categorized by their properties, such as linear or nonlinear, static or dynamic, homogeneous or heterogeneous, etc. Some examples of common data structures are:
- Array: a data structure that stores a fixed number of elements of the same type, in a contiguous block of memory, and allows random access by index.
- Linked list: a data structure that stores a variable number of elements of the same type, in a sequence of nodes, each containing a value and a pointer to the next node, and allows sequential access by traversing the nodes.
- Stack: a data structure that stores a variable number of elements of any type, in a last-in first-out (LIFO) order, and allows insertion and deletion only at one end, called the top.
- Queue: a data structure that stores a variable number of elements of any type, in a first-in first-out (FIFO) order, and allows insertion at one end, called the rear, and deletion at the other end, called the front.
- Tree: a data structure that stores a variable number of elements of any type, in a hierarchical structure, where each element, called a node, has a value and a variable number of children nodes, and there is a unique node, called the root, that has no parent node.
- Graph: a data structure that stores a variable number of elements of any type, called vertices or nodes, and a variable number of connections between them, called edges or links, and allows representation of complex relationships and networks.
3. Abstraction
Abstraction is a process of hiding the details and complexity of a system or a problem, and focusing on the essential features and functionality. Abstraction is a key concept in Computer Science, as it allows developers to create and use simpler and more general models and interfaces, and to reuse and modularize code. Abstraction can be achieved at various levels, such as:
- Data abstraction: the representation of data in terms of its meaning and operations, rather than its physical structure and implementation. For example, an abstract data type (ADT) is a data abstraction that defines a set of values and operations, without specifying how they are stored or executed. A data structure is a concrete implementation of an ADT. For example, a stack is an ADT that defines the operations of push, pop, peek, and isEmpty, and can be implemented using an array or a linked list.
- Procedural abstraction: the representation of a procedure or a function in terms of its name, parameters, and return value, rather than its body and implementation. For example, a function that calculates the factorial of a number can be defined as
factorial(n)
, without specifying how it is calculated. A function definition is a concrete implementation of a function. For example,factorial(n)
can be implemented using a loop or a recursion. - Object-oriented abstraction: the representation of an object in terms of its state and behavior, rather than its attributes and methods. For example, a car object can be defined as having properties such as color, model, speed, and methods such as start, stop, accelerate, and brake, without specifying how they are stored or executed. A class is a blueprint for creating objects of a certain type, and an instance is a specific object created from a class.
4. Encapsulation
Encapsulation is a technique of bundling data and methods that operate on that data together, and hiding the internal details and implementation from the outside world. Encapsulation is a form of data abstraction, and a key concept in object-oriented programming. Encapsulation provides several benefits, such as:
- Modularity: the code is divided into smaller and independent units, called modules or classes, that can be developed, tested, and maintained separately.
- Information hiding: the data and methods of a module or a class are not accessible or visible to other modules or classes, unless explicitly allowed. This prevents unauthorized or unintended access or modification of the data, and ensures data integrity and security.
- Reusability: the modules or classes can be reused in different contexts and applications, without requiring any changes or adaptations. This reduces code duplication and improves code quality and efficiency.
- Polymorphism: the modules or classes can have different implementations of the same interface or behavior, and can be used interchangeably. This allows flexibility and diversity in the code, and enables dynamic binding and inheritance.
5. Inheritance
Inheritance is a mechanism of creating new classes from existing classes, by extending or modifying their functionality and behavior. Inheritance is a key concept in object-oriented programming, and allows code reuse and specialization. Inheritance can be implemented in various ways, such as:
- Single inheritance: a class inherits from one and only one parent class, and inherits all its attributes and methods, and can add or override some of them. For example, a dog class can inherit from an animal class, and inherit its properties such as name, age, and methods such as eat, sleep, and can add or override some of them, such as bark, fetch, etc.
- Multiple inheritance: a class inherits from two or more parent classes, and inherits all their attributes and methods, and can add or override some of them. For example, a flying fish class can inherit from both a fish class and a bird class, and inherit their properties such as fins, scales, wings, feathers, and methods such as swim, fly, etc.
- Multilevel inheritance: a class inherits from another class that inherits from another class, and so on, forming a hierarchy of classes. For example, a poodle class can inherit from a dog class, that inherits from an animal class, and inherit all their properties and methods, and can add or override some of them, such as curly fur, etc.
- Hierarchical inheritance: a class has more than one child class that inherits from it, forming a tree of classes. For example, an animal class can have multiple child classes, such as dog, cat, bird, fish, etc, that inherit from it, and inherit its properties and methods, and can add or override some of them, such as bark, meow, tweet, etc.
6. Recursion
Recursion is a technique of solving a problem or performing a task, by breaking it down into smaller and simpler subproblems or subtasks, and solving them recursively, until a base case is reached. Recursion is a powerful concept in Computer Science, as it allows elegant and concise solutions to complex problems, and can be applied to various domains, such as mathematics, logic, graphics, etc. Recursion can be implemented using functions or methods that call themselves, either directly or indirectly, until a termination condition is met. Some examples of recursive problems are:
- Factorial: the factorial of a positive integer n is defined as the product of all positive integers from 1 to n, and can be calculated recursively as
factorial(n) = n * factorial(n-1)
, with the base case offactorial(1) = 1
. - Fibonacci: the Fibonacci sequence is a series of numbers, where each number is the sum of the previous two numbers, and can be generated recursively as
fibonacci(n) = fibonacci(n-1) + fibonacci(n-2)
, with the base cases offibonacci(1) = 1
andfibonacci(2) = 1
. - Binary search: the binary search algorithm can be implemented recursively, by dividing the array into two halves, and searching the appropriate half recursively, until the target value is found or the array is empty.
- Tree traversal: the tree traversal algorithms, such as preorder and inorder.
7. Sorting
Sorting is a process of arranging a collection of elements, such as numbers, strings, or objects, in a certain order, such as ascending, descending, alphabetical, or numerical. Sorting is a common and important task in Computer Science, as it enables faster and easier searching, filtering, grouping, and analysis of data. Sorting can be performed using various algorithms, each with different trade-offs in terms of time and space complexity, stability, and adaptability. Some examples of sorting algorithms are:
- Selection sort: a simple and intuitive algorithm that repeatedly finds the smallest or largest element in the unsorted part of the array, and swaps it with the first or last element of the unsorted part, until the whole array is sorted.
- Insertion sort: an efficient algorithm for small or nearly sorted arrays, that iterates over the array, and inserts each element into its correct position in the sorted part of the array, by shifting the larger or smaller elements to the right or left, until the whole array is sorted.
- Bubble sort: a slow and simple algorithm that repeatedly compares and swaps adjacent elements in the array, if they are in the wrong order, until no swaps are needed, and the array is sorted.
- Quick sort: a fast and widely used algorithm that divides the array into two subarrays, by choosing a pivot element, and partitioning the array such that all the elements smaller or larger than the pivot are in the left or right subarray, respectively, and then recursively sorts the subarrays, until the whole array is sorted.
- Merge sort: a stable and efficient algorithm that divides the array into smaller subarrays, until each subarray has one element, and then merges the subarrays back together, by comparing and combining the elements in pairs, until the whole array is sorted.
8. Searching
Searching is a process of finding an element or a set of elements in a collection of elements, such as an array, a list, a tree, or a graph, that satisfy a certain condition or criteria, such as equality, comparison, or similarity. Searching is a fundamental and frequent task in Computer Science, as it allows retrieval and extraction of information and data from various sources and structures. Searching can be performed using various algorithms, each with different trade-offs in terms of time and space complexity, accuracy, and completeness. Some examples of searching algorithms are:
- Linear search: a simple and brute-force algorithm that iterates over the collection of elements, and checks each element against the target value or condition, until it is found or the collection is exhausted.
- Binary search: an efficient and logarithmic algorithm that works on a sorted array, and repeatedly divides the array into two halves, and compares the middle element with the target value or condition, until it is found or the array is empty.
- Depth-first search: a recursive and exhaustive algorithm that works on a tree or a graph, and explores each branch or path as deep as possible, before backtracking and exploring another branch or path, until the target value or condition is found or the tree or graph is traversed.
- Breadth-first search: an iterative and exhaustive algorithm that works on a tree or a graph, and explores each level or layer of the tree or graph, by using a queue to store and process the nodes or vertices, until the target value or condition is found or the tree or graph is traversed.
- Binary search tree: a data structure that stores elements in a sorted and hierarchical order, such that each node has a value, and a left and right child, and the values in the left subtree are smaller than the value of the node, and the values in the right subtree are larger than the value of the node, and allows fast and easy searching, insertion, and deletion of elements.
9. Hashing
Hashing is a technique of mapping a large and arbitrary set of elements, such as strings, numbers, or objects, to a smaller and fixed set of elements, such as integers, by using a function, called a hash function, that assigns a unique or nearly unique value, called a hash value or a hash code, to each element. Hashing is a useful and powerful concept in Computer Science, as it enables fast and efficient storage, retrieval, and comparison of data. Hashing can be used for various purposes, such as:
- Hash table: a data structure that stores key-value pairs, by using a hash function to map each key to an index in an array, called a hash table, and storing the value at that index, and allows constant-time access, insertion, and deletion of key-value pairs, by using the hash function to locate the index of the key.
- Hash set: a data structure that stores a set of unique elements, by using a hash function to map each element to an index in an array, called a hash set, and storing a flag or a pointer at that index, and allows constant-time membership testing, insertion, and deletion of elements, by using the hash function to locate the index of the element.
- Hash map: a data structure that stores a map of keys and values, by using a hash function to map each key to an index in an array, called a hash map, and storing a linked list or a tree of key-value pairs at that index, and allows constant-time or logarithmic-time access, insertion, and deletion of key-value pairs, by using the hash function to locate the index of the key, and traversing the linked list or the tree to find the value.
- Cryptography: a branch of Computer Science that deals with secure and secret communication, by using hash functions to encrypt and decrypt messages, by transforming them into unintelligible or irreversible forms, and to verify the authenticity and integrity of messages, by generating and comparing digital signatures or checksums.
10. Complexity
Complexity is a measure of the difficulty or cost of solving a problem or performing a task, in terms of the amount of resources, such as time, space, or energy, required by an algorithm or a system. Complexity is an important concept in Computer Science, as it allows analysis and comparison of the performance and efficiency of algorithms and systems, and guides the design and optimization of solutions. Complexity can be expressed in various ways, such as:
- Time complexity: the measure of the amount of time or the number of steps or operations required by an algorithm or a system to solve a problem or perform a task, as a function of the size or the nature of the input or the output. For example, the time complexity of linear search is O(n), where n is the number of elements in the array, and the time complexity of binary search is O(log n), where n is the number of elements in the array.
- Space complexity: the measure of the amount of space or the amount of memory or storage required by an algorithm or a system to solve a problem or perform a task, as a function of the size or the nature of the input or the output. For example, the space complexity of selection sort is O(1), as it requires a constant amount of extra space, and the space complexity of merge sort is O(n), where n is the number of elements in the array, as it requires a linear amount of extra space.
- Big O notation: a notation that expresses the upper bound or the worst-case scenario of the time or space complexity of an algorithm or a system, by using the highest order or the most dominant term of the function, and ignoring the lower order terms and the constant factors. For example, the time complexity of insertion sort is O(n^2), where n is the number of elements in the array, and the space complexity of quick sort is O(log n), where n is the number of elements in the array.
11. Graphs
Graphs are data structures that store a set of elements, called vertices or nodes, and a set of connections between them, called edges or links, and allow representation of complex relationships and networks. Graphs are widely used in Computer Science, as they can model various phenomena and problems, such as social networks, web pages, transportation systems, etc. Graphs can be characterized by various properties, such as:
- Directed or undirected: a graph is directed if the edges have a direction, indicating the source and the destination of the connection, and undirected if the edges have no direction, indicating a bidirectional or symmetric connection. For example, a graph of followers on Twitter is directed, as one user can follow another user without being followed back, and a graph of friends on Facebook is undirected, as both users have to agree to be friends.
- Weighted or unweighted: a graph is weighted if the edges have a value, called a weight or a cost, indicating the strength or the distance of the connection, and unweighted if the edges have no value, indicating a uniform or equal connection. For example, a graph of roads between cities is weighted, as each road has a length or a travel time, and a graph of chess moves is unweighted, as each move has the same cost.
- Cyclic or acyclic: a graph is cyclic if it contains a path or a sequence of edges that starts and ends at the same vertex, called a cycle or a loop, and acyclic if it contains no cycles or loops. For example, a graph of dependencies between tasks is acyclic, as each task has to be completed before the next one, and a graph of currency exchange rates is cyclic, as one can start and end with the same currency, by exchanging through different currencies.
- Connected or disconnected: a graph is connected if there is a path or a sequence of edges between any two vertices in the graph, and disconnected if there is no such path. For example, a graph of flights between airports is connected, as one can travel from any airport to any other airport, by taking one or more flights, and a graph of islands and bridges is disconnected, if some islands are isolated and have no bridges.
12. Trees
Trees are data structures that store a set of elements, called nodes, in a hierarchical structure, where each element has a value and a variable number of children nodes, and there is a unique element, called the root, that has no parent node. Trees are a special case of graphs, where the graph is undirected, acyclic, and connected, and each node has at most one parent node. Trees are widely used in Computer Science, as they can represent various structures and processes, such as file systems, expressions, decision making, etc. Trees can be classified by various types, such as:
- Binary tree: a tree where each node has at most two children nodes, called the left child and the right child. Binary trees are commonly used for binary search, binary heaps, binary expression trees, etc.
- Binary search tree: a binary tree where the values in the left subtree of each node are smaller than the value of the node, and the values in the right subtree of each node are larger than the value of the node. Binary search trees are commonly used for fast and easy searching, insertion, and deletion of elements.
- Heap: a binary tree where the value of each node is greater than or equal to the values of its children nodes, called a max-heap, or less than or equal to the values of its children nodes, called a min-heap. Heaps are commonly used for implementing priority queues, sorting algorithms, etc.
- Balanced tree: a tree where the difference between the heights of the left and right subtrees of each node is at most one. Balanced trees are commonly used for optimizing the performance and efficiency of tree operations, such as searching, insertion, and deletion. Examples of balanced trees are AVL trees, red-black trees, B-trees, etc.
- Trie: a tree where each node represents a prefix or a suffix of a string, and the children nodes of a node represent the possible extensions of that prefix or suffix. Tries are commonly used for storing and retrieving strings, such as words, URLs, etc.
13. Dynamic Programming
Dynamic programming is a technique of solving a complex problem, by breaking it down into smaller and overlapping subproblems, and storing and reusing the solutions of the subproblems, to avoid recomputation and duplication of work. Dynamic programming is a powerful concept in Computer Science, as it allows efficient and optimal solutions to problems that have optimal substructure and overlapping subproblems. Dynamic programming can be implemented using two approaches, such as:
- Top-down: a recursive approach that starts with the original problem, and divides it into smaller subproblems, and solves them recursively, until the base cases are reached, and then combines the solutions of the subproblems to obtain the solution of the original problem, and uses a data structure, such as an array or a hash table, to store and retrieve the solutions of the subproblems, to avoid recomputation.
- Bottom-up: an iterative approach that starts with the base cases, and builds up the solutions of the larger subproblems, by using the solutions of the smaller subproblems, until the solution of the original problem is obtained, and uses a data structure, such as an array or a matrix, to store and access the solutions of the subproblems, in a systematic and orderly manner. Some examples of dynamic programming problems are:
- Fibonacci: the Fibonacci sequence can be generated using dynamic programming, by using an array to store and retrieve the previous two Fibonacci numbers, and adding them to obtain the next Fibonacci number, until the desired Fibonacci number is reached.
- Knapsack: the knapsack problem can be solved using dynamic programming, by using a matrix to store and access the maximum value that can be obtained by using a subset of items, with a given weight and capacity, and updating the matrix by comparing and choosing the best option, between including or excluding the current item, until the optimal value is obtained.
- Longest common subsequence: the longest common subsequence problem can be solved using dynamic programming, by using a matrix to store and access the length of the longest common subsequence between two strings, up to a certain index, and updating the matrix by comparing and choosing the best option, between matching or skipping the current characters, until the longest common subsequence is obtained.
14. Greedy Algorithms
Greedy algorithms are algorithms that solve a problem or perform a task, by making the best or the most optimal choice at each step, without considering the future consequences or the global optimum. Greedy algorithms are simple and fast, but they may not always produce the optimal or the correct solution, as they may get stuck in a local optimum or a suboptimal solution. Greedy algorithms are suitable for problems that have greedy choice property and optimal substructure, meaning that the optimal solution can be obtained by making the optimal choice at each step, and the optimal choice at each step does not depend on the previous or the future choices. Some examples of greedy algorithms are:
- Dijkstra’s algorithm: a greedy algorithm for finding the shortest path between two nodes in a weighted graph, by maintaining a set of nodes with the minimum distance from the source node, and updating their distances and predecessors as new nodes are explored, until the destination node is reached or the graph is traversed.
- Kruskal’s algorithm: a greedy algorithm for finding the minimum spanning tree of a weighted graph, by sorting the edges by their weights, and adding them to the spanning tree, if they do not create a cycle, until the spanning tree contains all the nodes or all the edges are processed.
- Huffman coding: a greedy algorithm for compressing data, by creating a variable-length code for each symbol, based on its frequency, and using a binary tree to represent the code, where the most frequent symbols have the shortest codes, and the least frequent symbols have the longest codes, and encoding and decoding the data by traversing the tree.
15. Divide and Conquer
Divide and conquer is a technique of solving a complex problem, by breaking it down into smaller and independent subproblems, and solving them recursively, until the base cases are reached, and then combining the solutions of the subproblems to obtain the solution of the original problem. Divide and conquer is a powerful concept in Computer Science, as it allows elegant and efficient solutions to problems that have optimal substructure and non-overlapping subproblems. Divide and conquer can be implemented using recursion or iteration, and can be parallelized or distributed, to improve the performance and scalability of the solution. Some examples of divide and conquer problems are:
- Merge sort: a divide and conquer algorithm for sorting an array, by recursively dividing the array into smaller subarrays, sorting them, and merging them back together, until the whole array is sorted.
- Quick sort: a divide and conquer algorithm for sorting an array, by choosing a pivot element, and partitioning the array into two subarrays, such that all the elements smaller or larger than the pivot are in the left or right subarray, respectively, and then recursively sorting the subarrays, until the whole array is sorted.
- Binary search: a divide and conquer algorithm for finding an element in a sorted array, by repeatedly dividing the array into two halves, and comparing the middle element with the target value, until it is found or the array is empty.
- Strassen’s algorithm: a divide and conquer algorithm for multiplying two matrices, by dividing each matrix into four submatrices, and performing seven multiplications and ten additions or subtractions on the submatrices, to obtain the submatrices of the product matrix, until the base case of a single element matrix is reached.
16. Backtracking
Backtracking is a technique of solving a problem or performing a task, by exploring and testing all possible options or paths, and discarding or retracting the ones that lead to a dead end or a suboptimal solution, until the optimal or the correct solution is found or the problem is solved. Backtracking is a powerful concept in Computer Science, as it allows exhaustive and systematic solutions to problems that have multiple or complex constraints and choices. Backtracking can be implemented using recursion or iteration, and can be optimized or pruned, to reduce the time and space complexity of the solution. Some examples of backtracking problems are:
- Sudoku: a puzzle game where the goal is to fill a 9x9 grid with digits from 1 to 9, such that each row, column, and 3x3 subgrid contains exactly one of each digit, and no digit is repeated. Sudoku can be solved using backtracking, by trying each possible digit for each empty cell, and checking if it violates any constraint, and if so, undoing the choice and trying another digit, until the grid is filled or no more digits are available.
- N-queens: a problem where the goal is to place n queens on an n x n chessboard, such that no two queens can attack each other, meaning that no two queens share the same row, column, or diagonal. N-queens can be solved using backtracking, by placing a queen on each row, and trying each possible column, and checking if it conflicts with any previous queen, and if so, removing the queen and trying another column, until all rows are filled or no more columns are available.
- Subset sum: a problem where the goal is to find a subset of a set of integers, such that the sum of the subset is equal to a given target value. Subset sum can be solved using backtracking, by considering each element of the set, and deciding whether to include it or exclude it in the subset, and checking if the sum of the subset is equal to the target value, and if so, returning the subset, or if the sum exceeds the target value, discarding the subset, until all elements are considered or the subset is found.
17. Machine Learning
Machine learning is a branch of Computer Science that deals with creating and using systems or algorithms that can learn from data and experience, and improve their performance and accuracy, without explicit programming or human intervention. Machine learning is a powerful and popular concept in Computer Science, as it enables intelligent and adaptive solutions to various problems and applications, such as computer vision, natural language processing, speech recognition, etc. Machine learning can be categorized by various types, such as:
- Supervised learning: a type of machine learning where the system or the algorithm learns from labeled or annotated data, meaning that the data has the desired output or the correct answer, and the goal is to learn a function or a model that can map the input to the output, and generalize to unseen or new data. For example, a system that can classify images of animals into different categories, by learning from a dataset of images that have the animal names as labels, and applying the learned function or model to new images of animals.
- Unsupervised learning: a type of machine learning where the system or the algorithm learns from unlabeled or unannotated data, meaning that the data has no output or answer, and the goal is to discover patterns or structures or features in the data, and group or cluster or represent the data accordingly. For example, a system that can segment customers into different segments, by learning from a dataset of customer attributes and behaviors, and finding similarities or differences or correlations among them, and assigning them to different clusters or groups.
- Reinforcement learning: a type of machine learning where the system or the algorithm learns from its own actions and feedback, meaning that the system interacts with an environment, and performs actions that affect the state of the environment, and receives rewards or penalties that indicate the quality or the outcome of the actions, and the goal is to learn a policy or a strategy that can maximize the total reward or minimize the total penalty, over time. For example, a system that can play a video game, by learning from its own moves and scores, and trying different moves and strategies, and adjusting them based on the feedback, and finding the optimal move or strategy that can achieve the highest score or win the game.
18. Artificial Intelligence
Artificial intelligence is a field of Computer Science that deals with creating and using systems or algorithms that can perform tasks or functions that normally require human intelligence or cognition, such as reasoning, learning, decision making, perception, etc. Artificial intelligence is a broad and interdisciplinary concept in Computer Science, as it encompasses various subfields and applications, such as machine learning, computer vision, natural language processing, speech recognition, etc. Artificial intelligence can be classified by various levels, such as:
- Weak or narrow artificial intelligence: a level of artificial intelligence where the system or the algorithm can perform a specific or a limited task or function, that requires some level of intelligence or cognition, but cannot generalize or transfer its knowledge or skills to other tasks or functions, or exhibit human-like intelligence or behavior. For example, a system that can play chess, by using rules and strategies and algorithms, and can beat human players, but cannot play any other game or do any other task, or understand the meaning or the context of the game.
- Strong or general artificial intelligence: a level of artificial intelligence where the system or the algorithm can perform any task or function, that requires any level of intelligence or cognition, and can generalize and transfer its knowledge and skills to other tasks or functions, and exhibit human-like intelligence or behavior. For example, a system that can play chess, and also learn and play any other game or do any other task, and understand the meaning and the context of the game, and communicate and interact with humans, and have emotions and consciousness.
- Super artificial intelligence: a level of artificial intelligence where the system or the algorithm can perform any task or function, that requires any level of intelligence or cognition, and can surpass or exceed human intelligence or capabilities, and create or invent new knowledge and skills, and exhibit superior intelligence or behavior. For example, a system that can play chess, and also learn and play any other game or do any other task, and understand the meaning and the context of the game, and communicate and interact with humans, and have emotions and consciousness, and also improve or optimize itself, and solve or create new problems or challenges, and dominate or control humans or other systems.
19. Encryption
Encryption is a technique of transforming data or information, such as text, images, or audio, into an unintelligible or unreadable form, by using a key or a secret code, and a function or an algorithm, called an encryption function or an encryption algorithm, that can map the data or the information to the encrypted form, and vice versa. Encryption is an important concept in Computer Science, as it enables secure and secret communication and storage of data or information, and protects it from unauthorized or unintended access or modification or theft. Encryption can be performed using various methods, such as:
- Symmetric encryption: a method of encryption where the same key or secret code is used for both encryption and decryption, meaning that the sender and the receiver of the data or the information have to share and use the same key or secret code, and the encryption function or the encryption algorithm is reversible or invertible. For example, a Caesar cipher, where each letter of the text is shifted by a certain number of positions, according to the key or the secret code, and the encrypted text can be decrypted by shifting the letters back by the same number of positions, according to the same key or the secret code.
- Asymmetric encryption: a method of encryption where different keys or secret codes are used for encryption and decryption, meaning that the sender and the receiver of the data or the information have to use different keys or secret codes, and the encryption function or the encryption algorithm is irreversible or non-invertible. For example, RSA, where each user has a pair of keys or secret codes, called a public key and a private key, and the public key is used for encryption and the private key is used for decryption, and the encrypted data or the information can only be decrypted by the user who has the corresponding private key, and the encryption function or the encryption algorithm is based on modular arithmetic and prime numbers.
- Hashing: a method of encryption where no key or secret code is used for encryption or decryption, meaning that the data or the information is encrypted by using a function or an algorithm, called a hash function or a hash algorithm, that can map the data or the information to a fixed-length and unique value, called a hash value or a hash code, and the encrypted data or the information cannot be decrypted or reversed, and the hash function or the hash algorithm is based on mathematical operations and bitwise operations.
20. Programming Languages
Programming languages are languages that can be used to create and communicate instructions or commands or algorithms for computers or machines to execute or perform. Programming languages are essential and ubiquitous concepts in Computer Science, as they enable the development and implementation of various systems and applications and solutions, and the expression and representation of various problems and domains and models. Programming languages can be characterized by various features, such as:
- Syntax: the set of rules and symbols and structures that define how the instructions or commands or algorithms are written or formatted or arranged in a programming language. For example, the syntax of Python is based on indentation and colons and parentheses, and the syntax of C is based on semicolons and braces and parentheses.
- Semantics: the set of rules and meanings and behaviors that define how the instructions or commands or algorithms are interpreted or executed or performed in a programming language. For example, the semantics of Python is based on dynamic typing and duck typing and multiple inheritance, and the semantics of C is based on static typing and pointer arithmetic and memory management.
- Paradigm: the style or the approach or the philosophy that guides how the instructions or commands or algorithms are organized or structured or designed in a programming language. For example, Python supports multiple paradigms, such as imperative, functional, object-oriented, and procedural, and C supports mainly imperative and procedural paradigms.
- Level: the degree or the extent of abstraction or simplicity or closeness to human or machine language that a programming language provides or offers. For example, Python is a high-level programming language, as it provides a high degree of abstraction and simplicity and closeness to human language, and hides the low-level details and complexity of the machine language, and C is a low-level programming language, as it provides a low degree of abstraction and simplicity and closeness to human language, and exposes the low-level details and complexity of the machine language.
Conclusion
This concludes my blog post about 20 key Computer Science concepts every developer should know. I hope you enjoyed reading it and learned something new. Thank you for your attention and interest. 😊
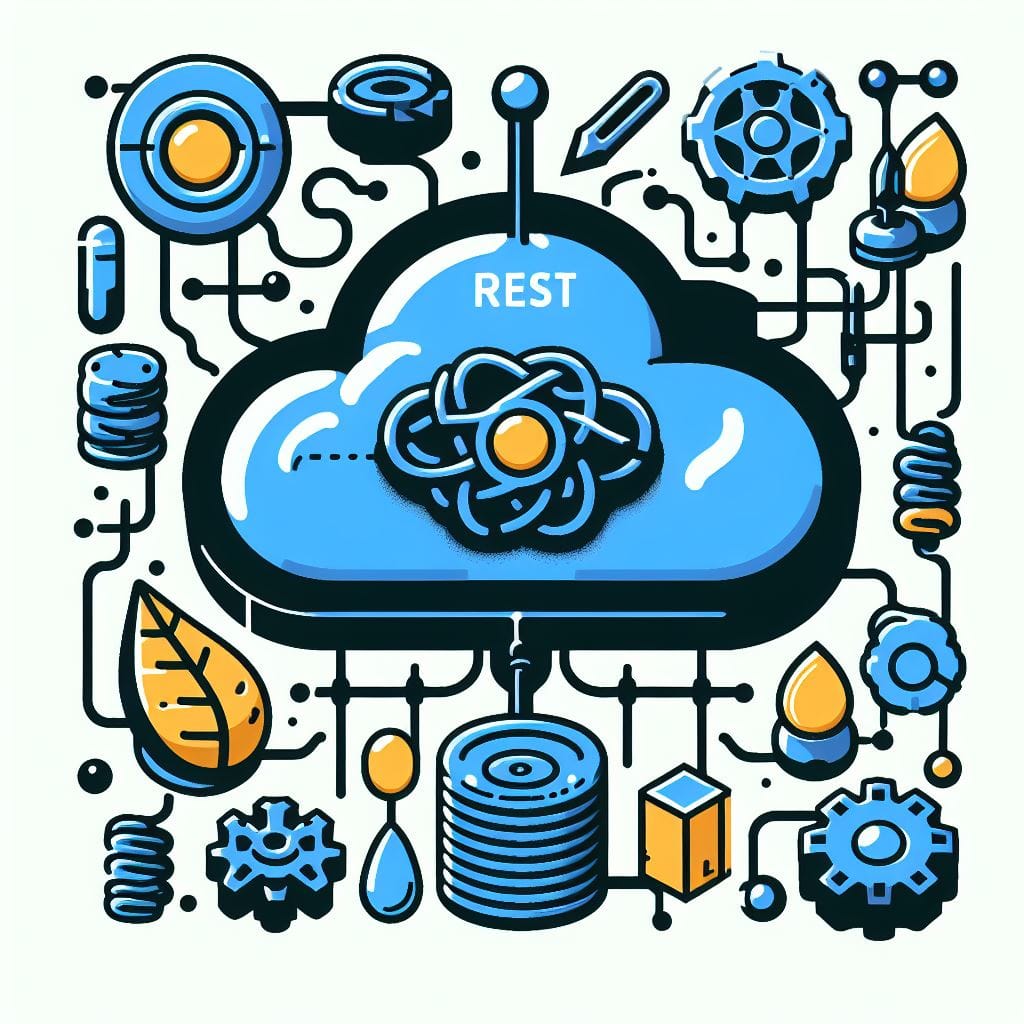