How a zero can change everything in Java (a puzzler)
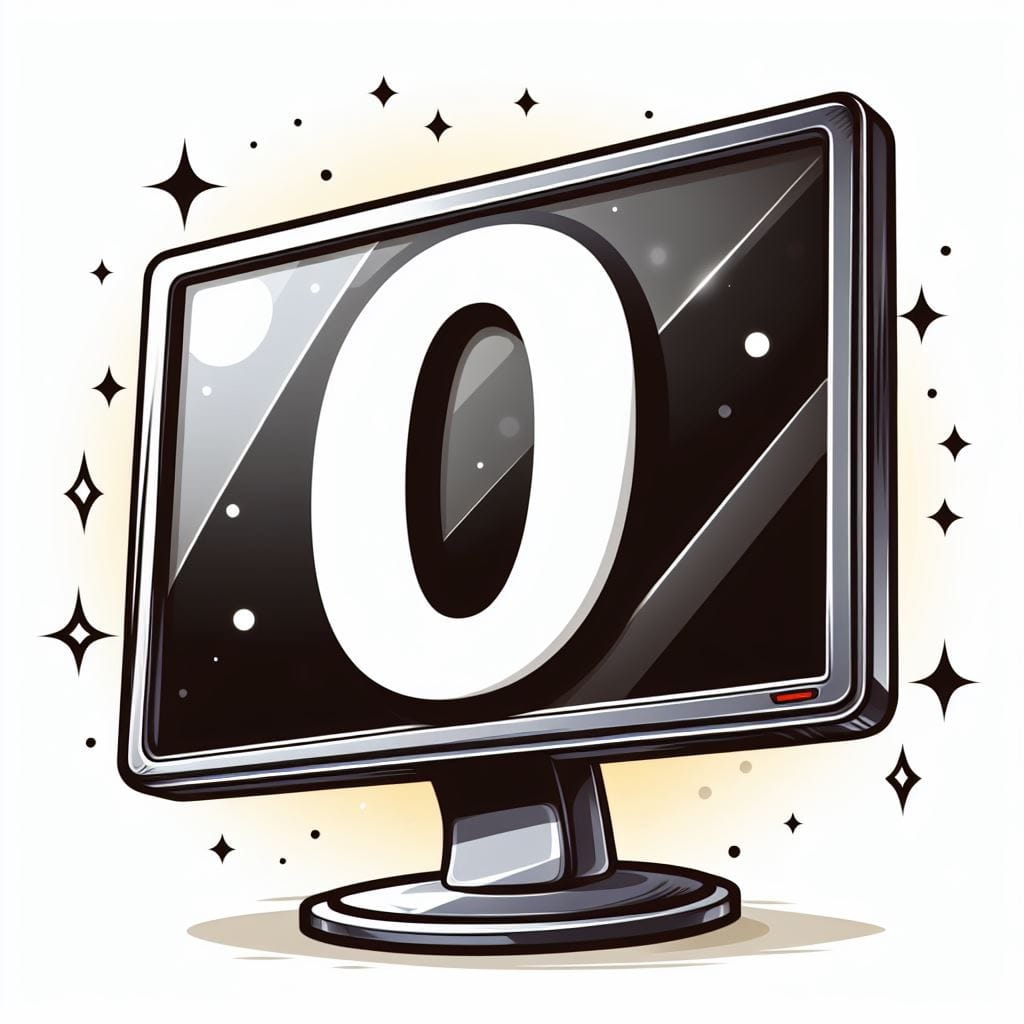
Java is a popular and widely used programming language, but it also has some quirks and surprises that can puzzle even experienced developers. In this blog post, we will explore one such puzzler: what does the following code print?
public class Puzzler {
public static void main(String[] args) {
int x = 010;
System.out.println(x);
}
}
Reveal answer
If you run this code, you will see that it prints 8
to the standard output. But why? Shouldn’t it print 10
, since that is the value we assigned to the variable x
?
If an integer literal starts with a zero, it is treated as an octal number
The answer lies in the way Java interprets integer literals. A literal is a fixed value that is written directly in the code, such as 10
, true
, or "Hello"
. Java has different rules for different types of literals, and one of them is that if an integer literal starts with a zero, it is treated as an octal number.
Octal is a base-8 number system, which means it uses eight digits from 0 to 7. To convert an octal number to a decimal number, we need to multiply each digit by a power of 8, starting from the rightmost digit.
For example, the octal number 010
is equivalent to the decimal number 1 * 8^1 + 0 * 8^0 = 8 + 0 = 8
.
What if you use parseInt?
Let's try it! Let's see the code below:
public class Puzzler {
public static void main(String[] args) {
int x = Integer.parseInt("010");
System.out.println(x);
}
}
Integer's parseInt method strips out any leading zeroes before parsing, and outputs a 10
.
Conclusion
To avoid this confusion, we can use decimal literals (without leading zeros) for integer values, unless we explicitly want to use octal, hexadecimal, or binary literals.
This puzzler is a good example of how Java can sometimes behave in unexpected ways, and why we should always be careful and attentive when writing and reading code.
If you'd like to learn more useful to know puzzlers, check them out below! 😄
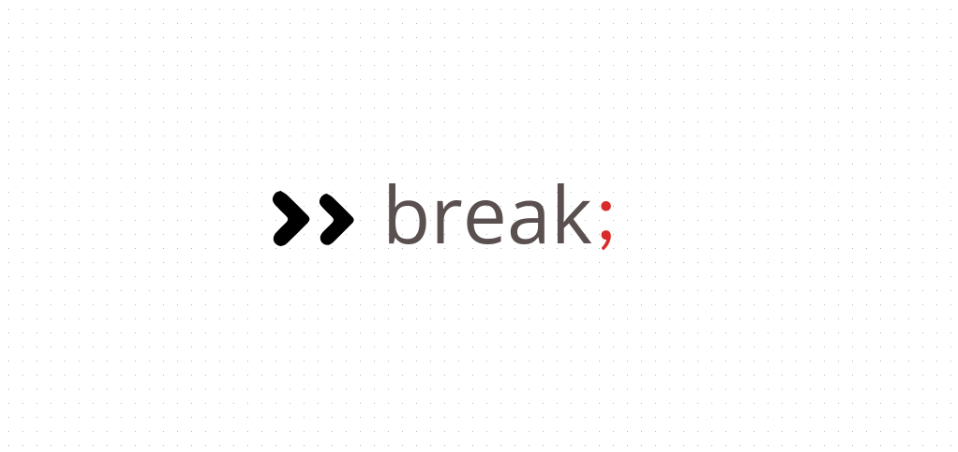
Things you might like
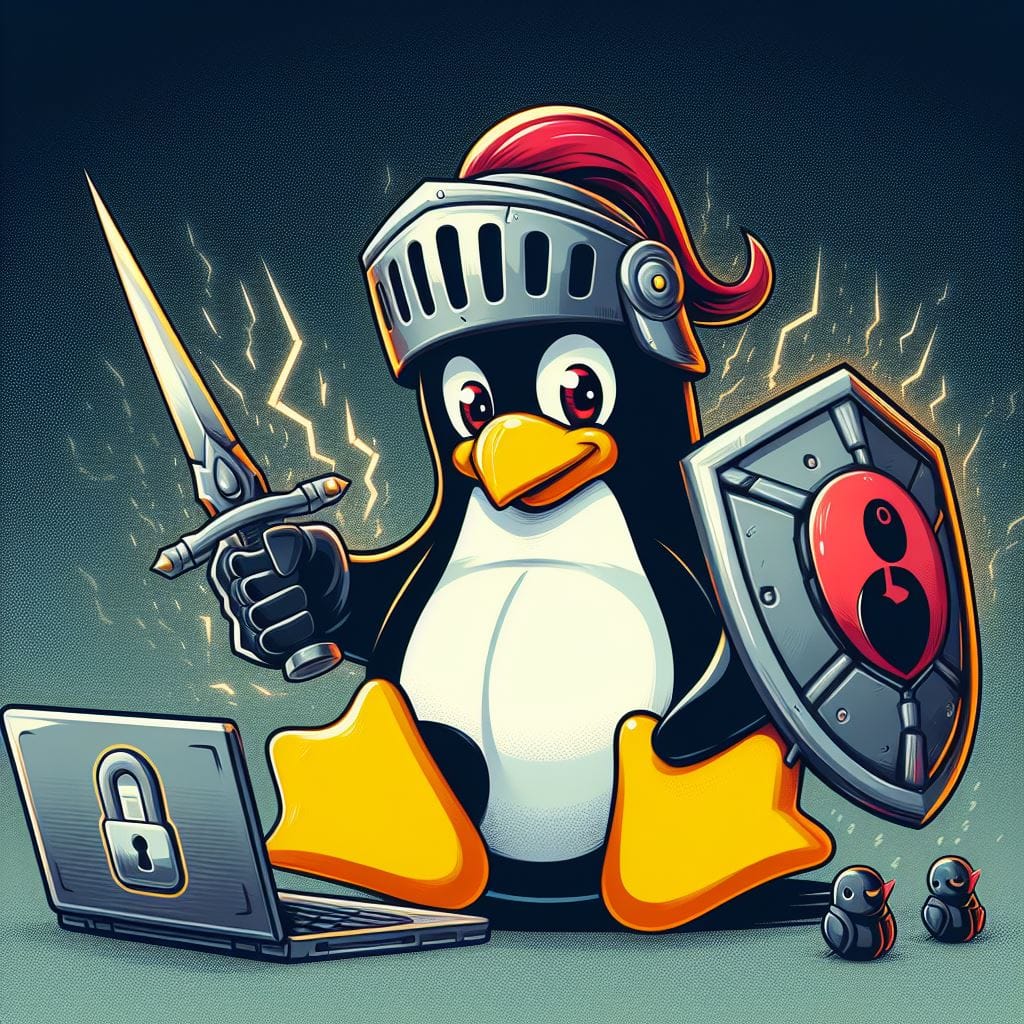
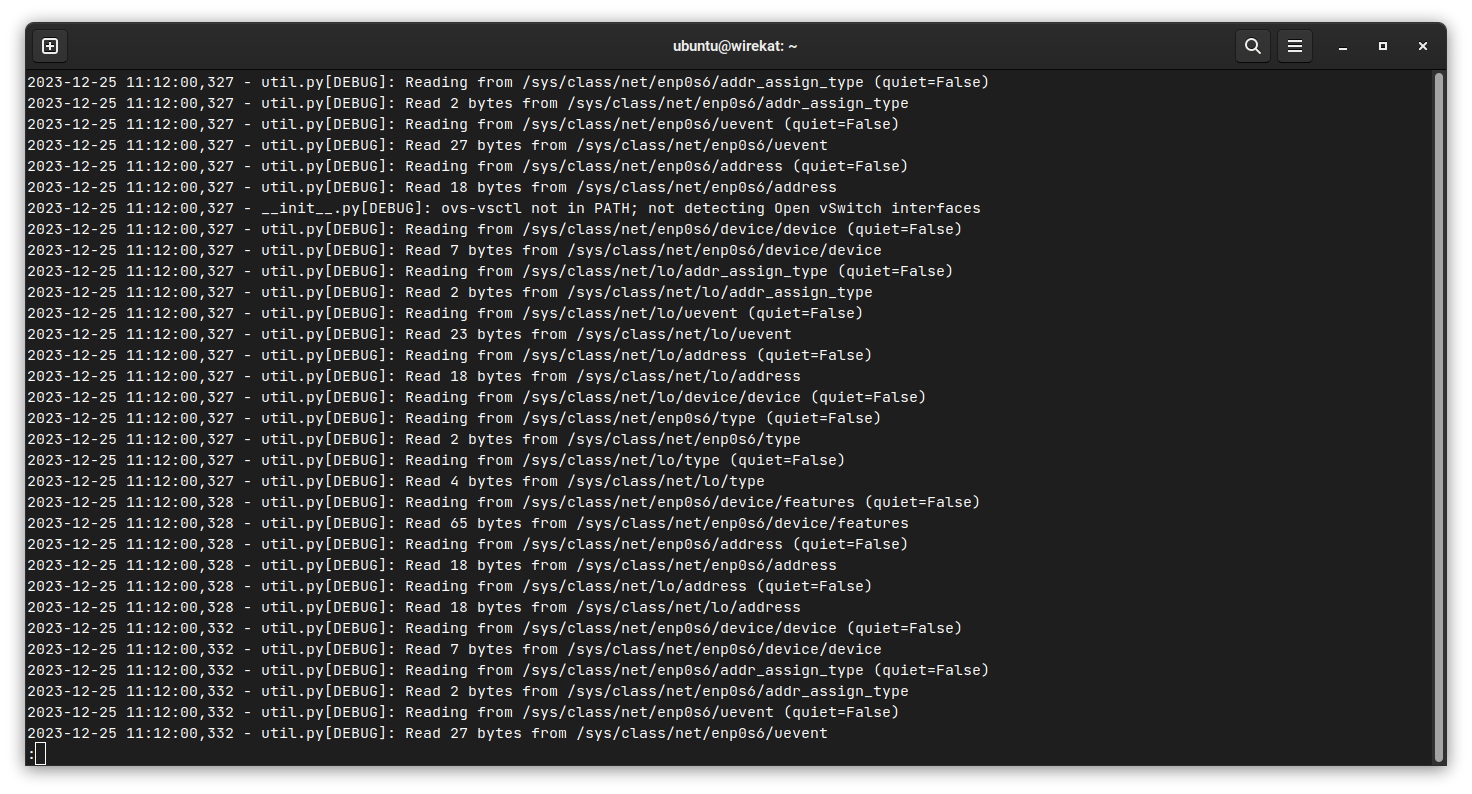