Do you know this Java puzzler about missing breaks in switches?
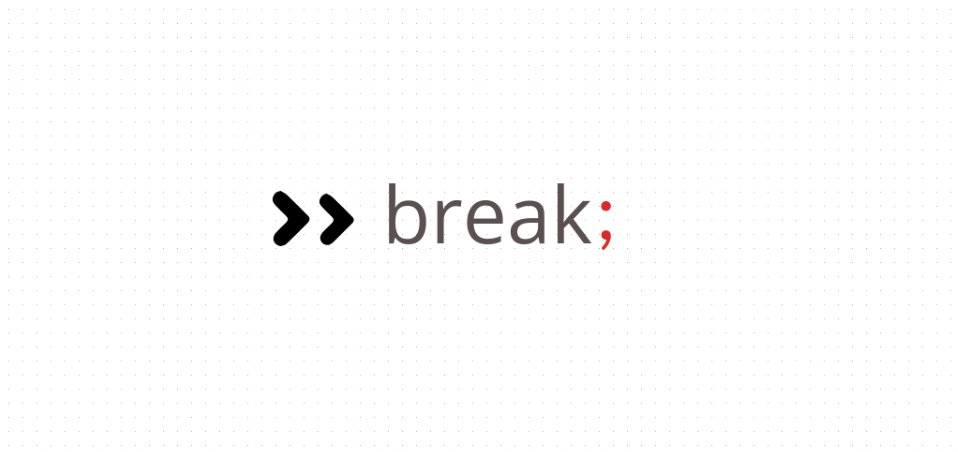
Java is a popular programming language that has many features and quirks. One of them is the switch statement, which allows you to execute different blocks of code based on the value of an expression.
For example, you can use a switch statement to print a message depending on the day of the week:
int day = 3; // Wednesday
switch (day) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
case 3:
System.out.println("Wednesday");
break;
case 4:
System.out.println("Thursday");
break;
case 5:
System.out.println("Friday");
break;
case 6:
System.out.println("Saturday");
break;
case 7:
System.out.println("Sunday");
break;
default:
System.out.println("Invalid day");
}
This code will print “Wednesday” as expected. However, what if we forget to add the break statement after each case? What will happen then?
int day = 3; // Wednesday
switch (day) {
case 1:
System.out.println("Monday");
case 2:
System.out.println("Tuesday");
case 3:
System.out.println("Wednesday");
case 4:
System.out.println("Thursday");
case 5:
System.out.println("Friday");
case 6:
System.out.println("Saturday");
case 7:
System.out.println("Sunday");
default:
System.out.println("Invalid day");
}
Do you see the mistake?
You might think that this code will print “Wednesday” and then stop, but that is not the case.
In fact, this code will print all the messages from “Wednesday” to “Invalid day”! This is because without the break statement, the execution will fall through to the next case, until it reaches the end of the switch statement or a break statement. This is a common source of bugs and confusion in Java, and it is often considered a bad practice to omit the break statement in a switch statement.
The solution
So, how can we avoid this problem? One way is to always use the break statement after each case, unless you want the fall through behavior intentionally.
Another way is to use an enum instead of an int for the day variable, and use an enhanced switch statement, which is a new feature introduced in Java 12. The enhanced switch statement does not require the break statement, and it will throw a compiler error if you try to fall through to another case. For example:
enum Day {MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY}
Day day = Day.WEDNESDAY;
switch (day) {
case MONDAY -> System.out.println("Monday");
case TUESDAY -> System.out.println("Tuesday");
case WEDNESDAY -> System.out.println("Wednesday");
case THURSDAY -> System.out.println("Thursday");
case FRIDAY -> System.out.println("Friday");
case SATURDAY -> System.out.println("Saturday");
case SUNDAY -> System.out.println("Sunday");
default -> System.out.println("Invalid day");
}
This code will print “Wednesday” and nothing else, as expected. If you try to remove the arrow (->
) after any case, you will get a compiler error saying “missing default label”. This way, you can avoid the accidental fall through problem and write more concise and clear code.
Conclusion
I hope you enjoyed this Java puzzler and learned something new. If you have any questions or comments, please leave them below.
If you want to learn about another simple yet quite interesting Java puzzler, check them out below!
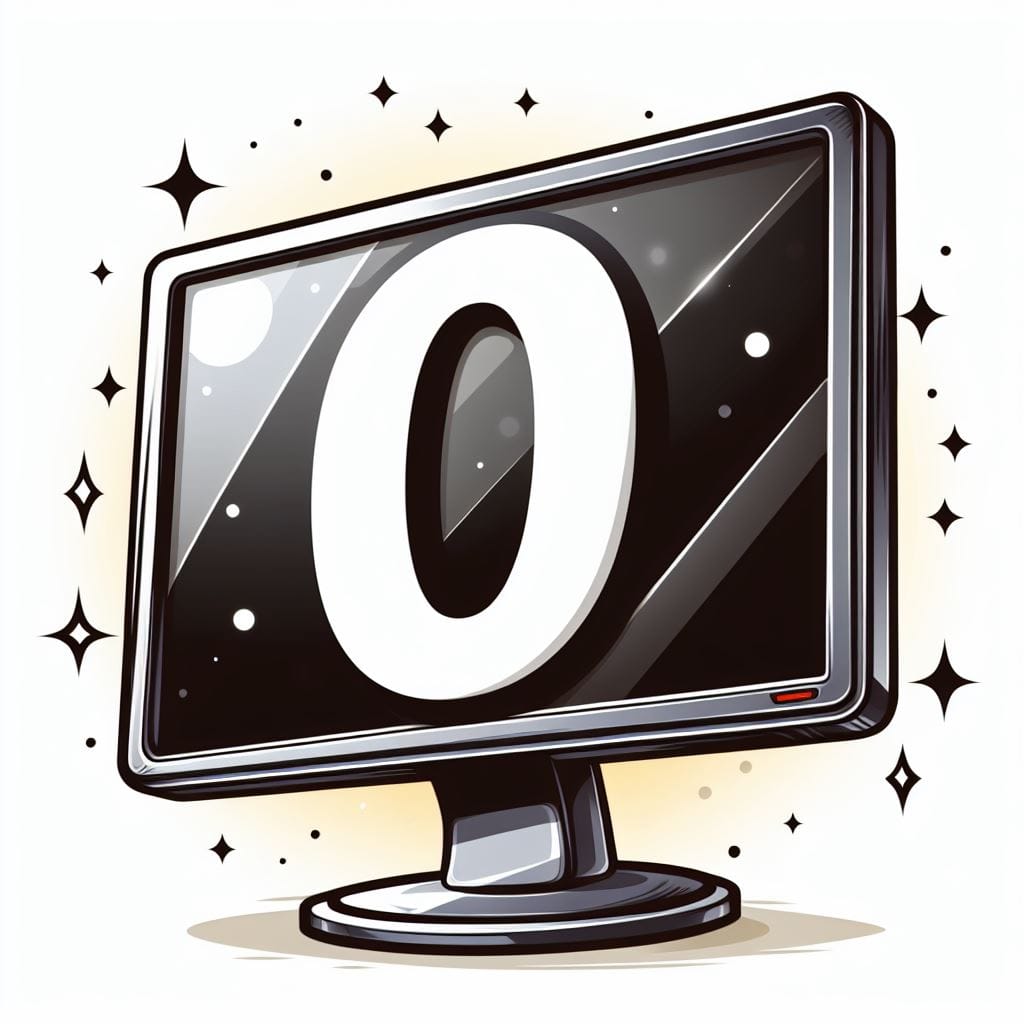