Logging in Java/Spring Boot - Best Practices and Tutorial
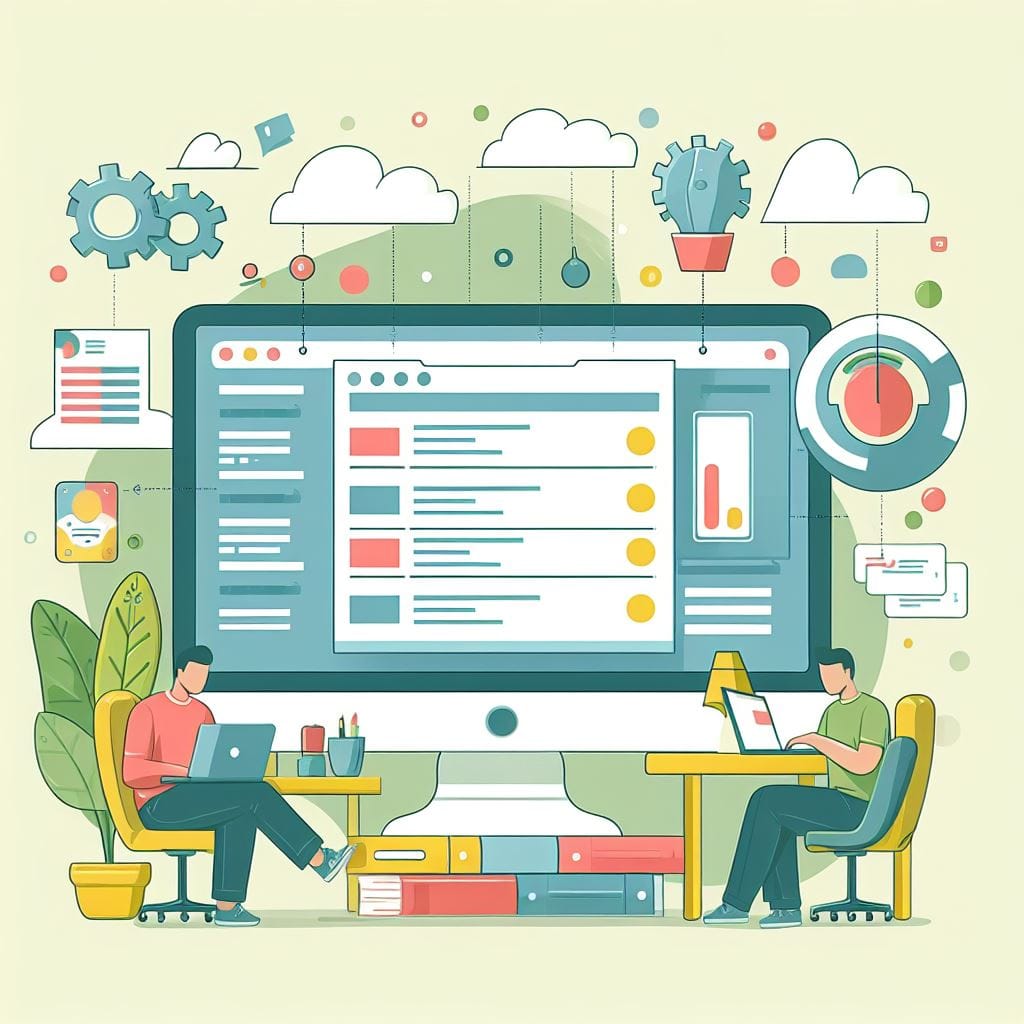
Logging is an essential part of any software development process. Logging is the act of recording events or messages that occur during the execution of a program. Logging can help developers to debug, monitor, and troubleshoot their applications, as well as provide valuable insights into the performance, behavior, and usage of their systems.
However, logging is not as simple as printing some text to the console or a file. Logging requires careful planning, design, and implementation to ensure that it is effective, efficient, and consistent. In this article, we will discuss some of the best practices for logging in Spring Boot applications, as well as provide a comprehensive tutorial on how to use the popular logging framework Logback with Spring Boot.
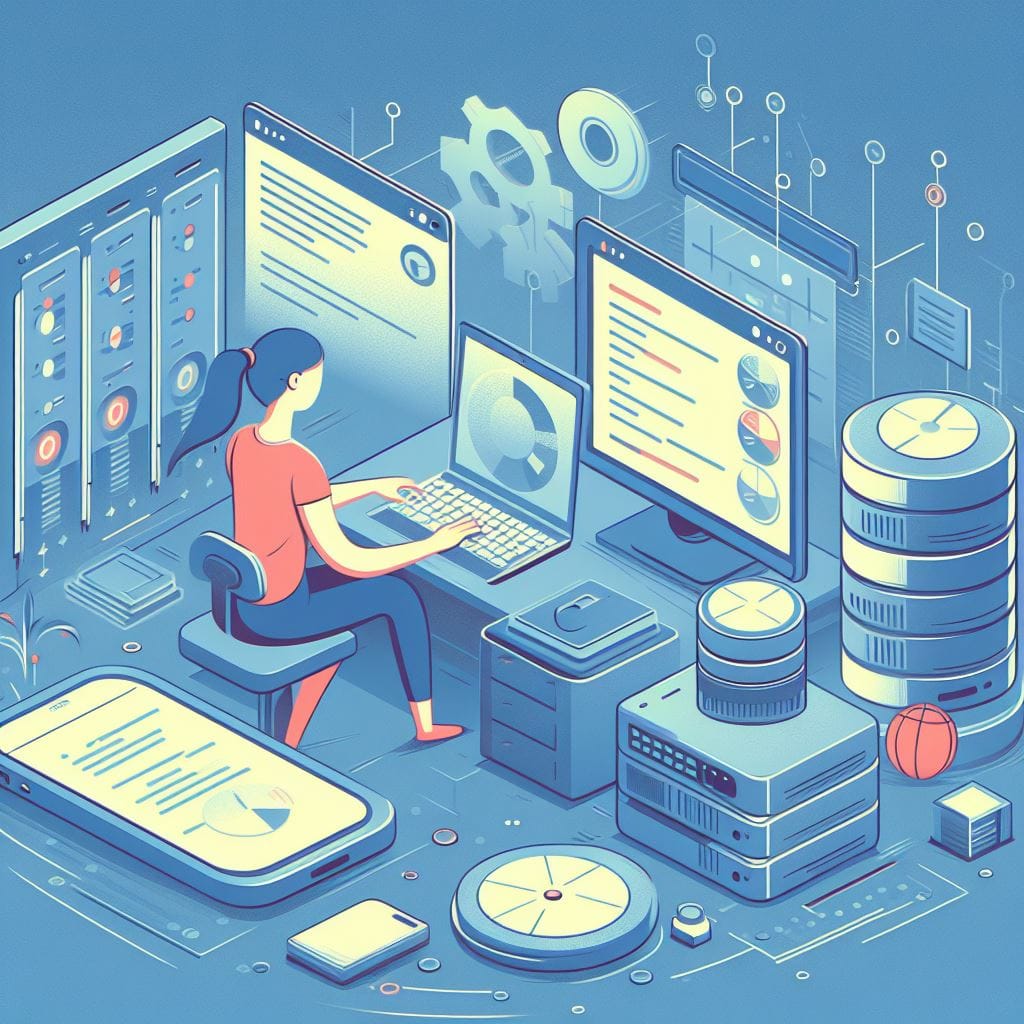
Best Practices for Logging
Logging is not a one-size-fits-all solution. Different applications may have different logging requirements and preferences. However, there are some general principles and guidelines that can help developers to achieve better logging quality and consistency. Here are some of the best practices for logging:
- Use a logging framework. A logging framework is a library or tool that provides a standardized and configurable way of logging messages. A logging framework can handle various aspects of logging, such as formatting, filtering, routing, and storing the log messages. A logging framework can also provide features such as log levels, appenders, layouts, and patterns. Using a logging framework can make logging easier, more flexible, and more consistent across different modules and environments. Some of the popular logging frameworks for Java are Logback, Log4j, and SLF4J.
- Use appropriate log levels. Log levels are a way of categorizing the log messages according to their severity or importance. Log levels can help developers to control the amount and detail of the log output, as well as filter and prioritize the log messages. Log levels can also help users and operators to identify and troubleshoot issues more quickly and effectively. The common log levels are:
- TRACE: The most detailed and verbose level of logging. TRACE messages are usually used for debugging purposes, to track the flow and state of the program. TRACE messages should only be enabled in development or testing environments, as they can generate a lot of noise and overhead in production.
- DEBUG: A less detailed level of logging than TRACE. DEBUG messages are also used for debugging purposes, to provide more information about the program logic and behavior. DEBUG messages can be useful for developers to diagnose and fix problems, but they should also be disabled in production, as they can still produce a lot of output and affect performance.
- INFO: A general level of logging that provides informative and useful messages about the program. INFO messages are used to report the normal and expected events and operations of the program, such as startup, shutdown, configuration, status, etc. INFO messages can be helpful for users and operators to monitor and understand the program, and they should be enabled in production, unless they are too frequent or redundant.
- WARN: A higher level of logging that indicates a potential problem or issue. WARN messages are used to report the abnormal or unexpected events and conditions of the program, such as errors, failures, violations, etc. WARN messages can alert users and operators to take preventive or corrective actions, and they should always be enabled in production, unless they are false positives or irrelevant.
- ERROR: The highest level of logging that indicates a serious problem or issue. ERROR messages are used to report the critical and fatal errors and exceptions of the program, such as crashes, data loss, corruption, etc. ERROR messages can indicate that the program is unable to function properly or at all, and they should always be enabled in production, as they require immediate attention and resolution.
- Use descriptive and consistent messages. The content and format of the log messages are also important for logging quality and consistency. Log messages should be descriptive and informative, providing enough detail and context to understand the event or issue. Log messages should also be consistent and follow a standard pattern or convention, such as using the same date and time format, delimiter, prefix, suffix, etc. Log messages should also avoid using ambiguous or vague terms, such as “error”, “failed”, “success”, etc., without specifying the cause, effect, or action. Log messages should also avoid using sensitive or confidential information, such as passwords, tokens, personal data, etc., as they can pose security and privacy risks.
- Use structured and machine-readable formats. The format and structure of the log messages are also important for logging efficiency and usability. Log messages should be structured and machine-readable, using a common and well-defined format, such as JSON, XML, CSV, etc. Structured and machine-readable log messages can make logging more scalable, reliable, and interoperable, as they can be easily parsed, processed, and transmitted by various tools and systems. Structured and machine-readable log messages can also make logging more searchable, analyzable, and actionable, as they can be easily queried, filtered, aggregated, and visualized by various tools and platforms.
- Use appropriate appenders and destinations. The appender and destination of the log messages are also important for logging performance and availability. The appender and destination are the components or mechanisms that handle the output and storage of the log messages. The appender and destination can vary depending on the type, format, and location of the log output, such as console, file, database, network, etc. The appender and destination should be appropriate and suitable for the logging requirements and preferences of the application, such as the volume, frequency, and retention of the log messages. The appender and destination should also be reliable and resilient, ensuring that the log messages are not lost, corrupted, or delayed.
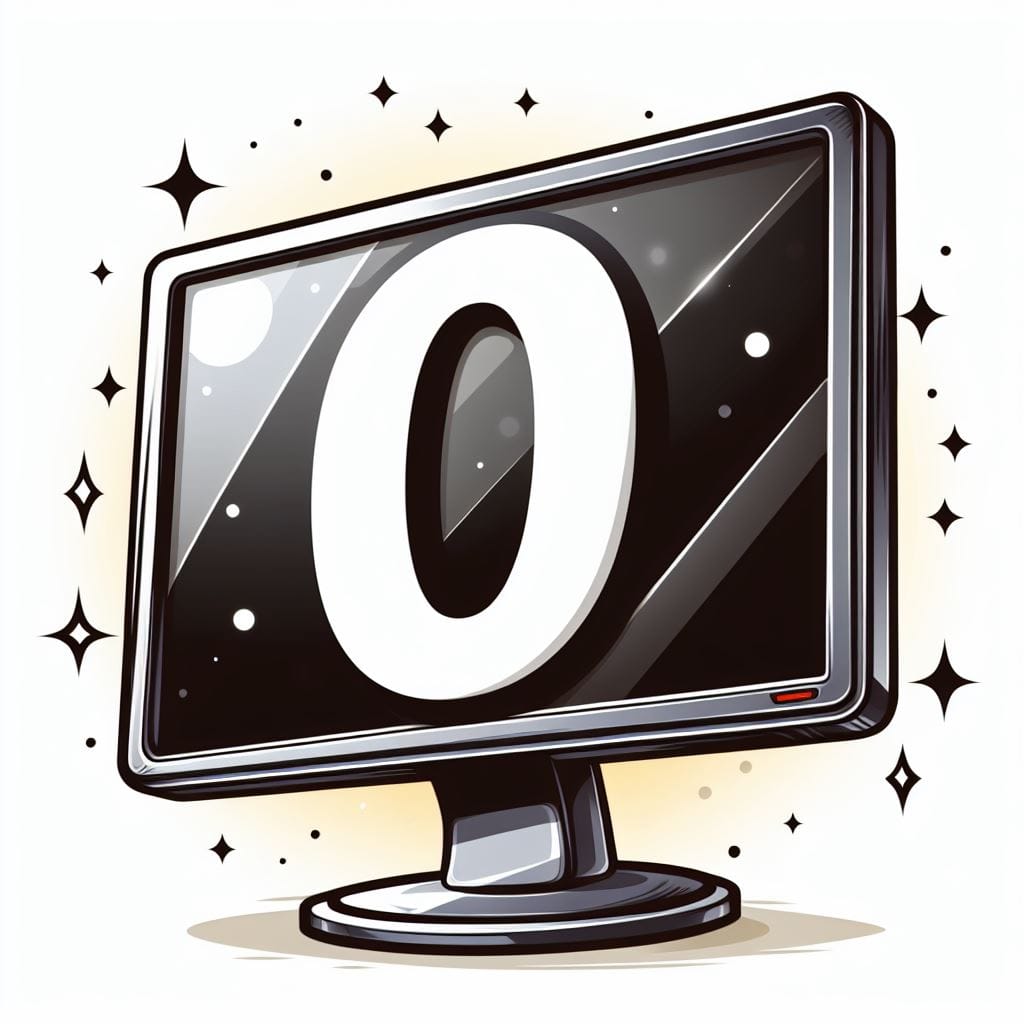
The default logging behavior in Spring Boot
Before we customize and configure the logging behavior of our application, it is useful to understand the default logging behavior that Spring Boot provides. Spring Boot uses a sensible and opinionated logging configuration that works well for most applications. The default logging behavior includes the following aspects:
- The default logging framework is Logback, which is a successor of Log4j and provides a simple and powerful way of logging messages.
- The default logging destination is the console, which means that the log messages are printed to the standard output stream (System.out) or the standard error stream (System.err) depending on the log level.
- The default logging file or path is not set, which means that the log messages are not written to any file or folder. However, we can set the logging.file.name or logging.file.path properties to enable file output, as will be explained below.
- The default logging format is color-coded and structured, which means that the log messages are displayed and organized using various elements and symbols, such as date, time, level, logger, message, etc. The log messages also use different colors to indicate the log level, such as red for ERROR, yellow for WARN, green for INFO, etc.
- The default logging pattern is “%d{yyyy-MM-dd HH:mm:ss.SSS} [%thread] %-5level %logger{36} - %msg%n”, which means that the log messages follow a standard template that consists of the following elements:
- %d{yyyy-MM-dd HH:mm:ss.SSS}: The date and time of the log message, in the format yyyy-MM-dd HH:mm:ss.SSS, such as 2024-02-10 11:59:13.123
- [%thread]: The thread name of the log message, in square brackets […], such as [main]
- %-5level: The log level of the log message, in uppercase letters, padded with spaces to 5 characters, such as INFO , DEBUG, WARN
- %logger{36}: The logger name of the log message, truncated to 36 characters, such as com.example.App, org.springframework.boot.web.embedded.tomcat.TomcatWebServer
- : A dash symbol - to separate the elements
- %msg: The log message itself, which can be any text or object, such as Application started successfully
- %n: A newline character \n to end the line
- The default logging level is INFO, which means that the log messages with the INFO level or higher (WARN and ERROR) are outputted, while the log messages with the lower levels (DEBUG and TRACE) are ignored. However, we can change the logging level by using the logging.level property or the --debug or --trace arguments, as we saw in the previous steps.
- The default logging rotation and compression are not enabled, which means that the log messages are not archived or compressed after a certain time or size. However, we can enable logging rotation and compression by using the logging.file.max-size, logging.file.max-history, and logging.file.total-size-cap properties, as we will see in the next steps.
Tutorial on Logging with Logback and Spring Boot
In this tutorial, we will demonstrate how to use the logging framework Logback with Spring Boot, a popular framework for building Java applications. Logback is a successor of Log4j, and it is the default logging framework for Spring Boot. Logback provides a simple and powerful way of logging messages, using various components such as loggers, appenders, layouts, and filters. Logback also supports various features such as log levels, patterns, variables, and profiles.
To follow this tutorial, you will need:
- Java 11 or higher
- Maven 3.6 or higher
- Spring Boot 2.5 or higher
Step 1: Create a Spring Boot project
The first step is to create a Spring Boot project using Maven. You can use the Spring Initializr website to generate the project.
Next, we need to add the Spring Boot dependencies and plugins to the pom.xml
file. We will also add the Logback Classic dependency, which is the core module of Logback that provides the logging functionality.
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-classic</artifactId>
<version>1.2.6</version>
</dependency>
Please look up the most current version instead
Step 2: Create a Spring Boot application class
The next step is to create a Spring Boot application class that will run the application and initialize the logging system. We will use the @SpringBootApplication
annotation to mark the class as a Spring Boot application, and the SpringApplication.run()
method to launch the application. We will also use the LoggerFactory
class to obtain a logger instance for the class, and the log.info()
method to log a message at the INFO level. The application class should look like this:
package com.example;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class App {
private static final Logger log = LoggerFactory.getLogger(App.class);
public static void main(String[] args) {
SpringApplication.run(App.class, args);
log.info("Application started successfully");
}
}
Alternatively if you use Lombok, you can append a @Slf4j
annotation and use log.*
the same way.
The application will log various messages at different levels, such as INFO, DEBUG, and WARN. The log messages also follow a standard pattern, which consists of the following elements:
- The timestamp of the log message, in the format
HH:mm:ss.SSS
- The thread name of the log message, in square brackets
[...]
- The log level of the log message, in uppercase letters
INFO
,DEBUG
,WARN
, etc. - The logger name of the log message, which is usually the fully qualified class name
com.example.App
,org.springframework.boot.web.embedded.tomcat.TomcatWebServer
, etc. - The log message itself, which can be any text or object
Starting App using Java 11.0.12 on copilot.local with PID 12345
,Tomcat started on port(s): 8080 (http) with context path ''
, etc.
This is the default logging configuration and behavior of Spring Boot and Logback. However, we can customize and configure various aspects of logging, such as the log level, the log pattern, the log destination, etc. We will see how to do that in the next steps.
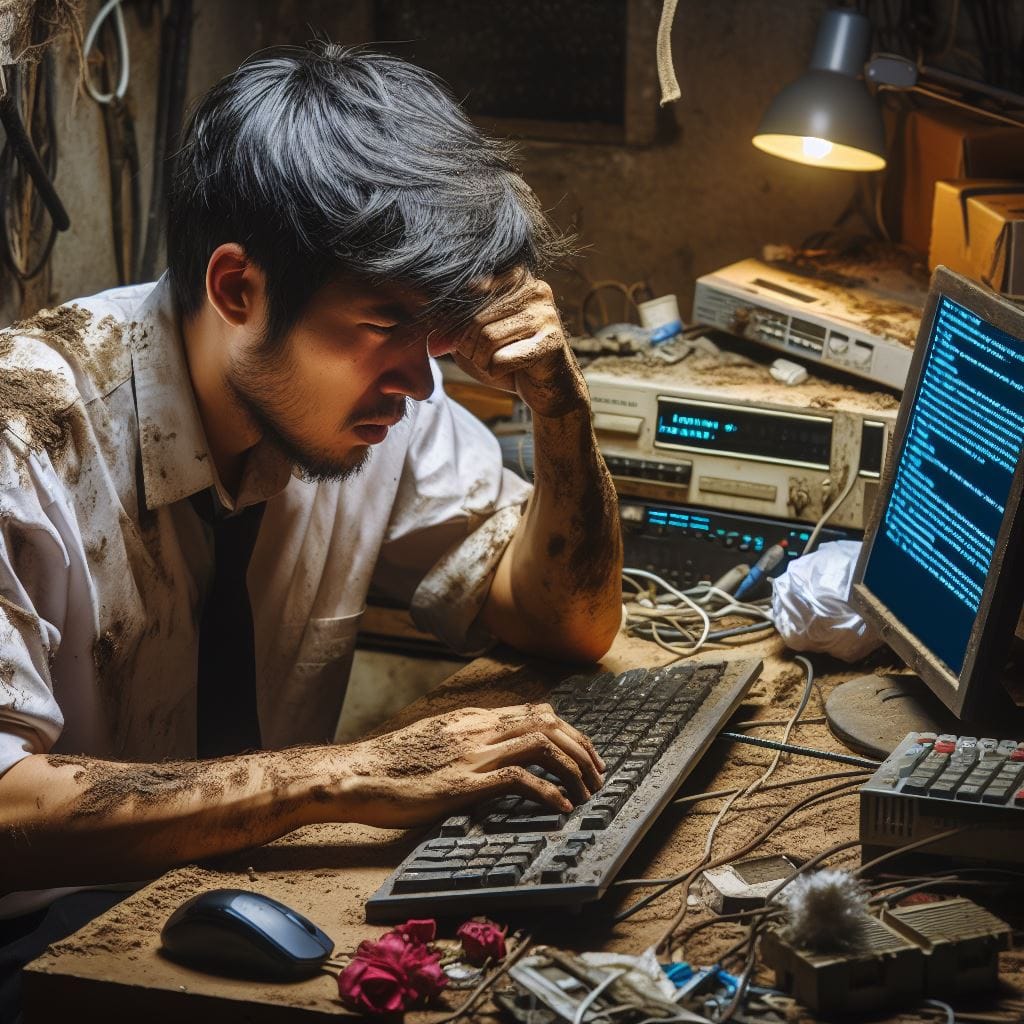
Step 3: Control log levels in the application.yml file
One of the ways to control the log levels of the application is to use the application.yml
file, which is a configuration file that Spring Boot uses to load various properties and settings. The application.yml
file is located in the src/main/resources
folder of the project, and it can be created if it does not exist.
To control the log levels of the application, we can use the logging.level
property, which allows us to specify the log level for a specific logger or a package. The syntax of the property is:
logging.level.<logger-name-or-package>: <log-level>
For example, if we want to set the log level of the root logger (which is the parent of all other loggers) to WARN, we can use the following property:
logging.level.root: WARN
If we want to set the log level of the com.example
package (which is the base package of our application) to DEBUG, we can use the following property:
logging.level.com.example: DEBUG
If we want to set the log level of the org.springframework
package (which is the base package of the Spring framework) to INFO, we can use the following property:
logging.level.org.springframework: INFO
We can also use the logging.level.*
property to set the log level for all other loggers that are not explicitly specified. For example, if we want to set the default log level to ERROR, we can use the following property:
logging.level.*: ERROR
The log levels that we can use are the same as the ones we discussed in the previous section: TRACE, DEBUG, INFO, WARN, and ERROR. The log levels are hierarchical, meaning that a higher level includes all the lower levels. For example, if we set the log level to WARN, it will also include ERROR messages, but not INFO, DEBUG, or TRACE messages.
The log levels that we specify in the application.yml
file will override the default log levels that are configured by Spring Boot and Logback. However, we can also use the spring.profiles.active
property to activate different log levels for different profiles or environments. For example, if we want to use different log levels for development and production profiles, we can use the following properties:
spring.profiles.active: dev
---
spring.profiles: dev
logging.level.root: DEBUG
---
spring.profiles: prod
logging.level.root: WARN
The ---
symbol is used to separate different sections or documents in the application.yml
file. The spring.profiles.active
property specifies the active profile for the application, which is dev
in this case. The spring.profiles
property specifies the profile for each section, which can be dev
or prod
in this case. The logging.level.root
property specifies the log level for the root logger for each profile, which is DEBUG
for dev
and WARN
for prod
in this case.
To run the application with a specific profile, we can use the following command:
mvn spring-boot:run -Dspring-boot.run.profiles=prod
This will run the application with the prod
profile, which will use the log level of WARN
for the root logger.
Step 4: Configure logging format in the application.yml file
Another way to customize the logging configuration of the application is to use the application.yml
file to configure the logging format, which is the way the log messages are displayed and structured. The logging format can affect the readability, usability, and interoperability of the log messages.
To configure the logging format of the application, we can use the logging.pattern
property, which allows us to specify the pattern or template for the log messages. The syntax of the property is:
logging.pattern.<console-or-file>: <pattern>
The <console-or-file>
parameter specifies the destination of the log messages, which can be either console
or file
. The <pattern>
parameter specifies the pattern or template for the log messages, which can be a combination of various elements or tokens, such as date, time, level, logger, message, etc. The pattern can also include various symbols or characters, such as spaces, commas, brackets, etc.
For example, if we want to change the pattern for the console output, we can use the following property:
logging.pattern.console: "%d{yyyy-MM-dd HH:mm:ss.SSS} [%t] %-5level %logger{36} - %msg%n"
This will change the pattern for the console output to include the following elements:
%d{yyyy-MM-dd HH:mm:ss.SSS}
: The date and time of the log message, in the formatyyyy-MM-dd HH:mm:ss.SSS
, such as2024-02-10 11:59:13.123
[%t]
: The thread name of the log message, in square brackets[...]
, such as[main]
%-5level
: The log level of the log message, in uppercase letters, padded with spaces to 5 characters, such asINFO
,DEBUG
,WARN
%logger{36}
: The logger name of the log message, truncated to 36 characters, such ascom.example.App
,org.springframework.boot.web.embedded.tomcat.TomcatWebServer
-
: A dash symbol-
to separate the elements%msg
: The log message itself, which can be any text or object, such asApplication started successfully
%n
: A newline character\n
to end the line
The result of this pattern will look like this:
2024-02-10 11:59:13.123 [main] INFO com.example.App - Application started successfully
If we want to change the pattern for the file output, we can use the following property:
logging.pattern.file: "%d{ISO8601} [%t] %-5level %logger{36} - %msg%n"
This will change the pattern for the file output to include the following elements:
%d{ISO8601}
: The date and time of the log message, in the ISO 8601 format, such as2024-02-10T11:59:13.123+00:00
[%t]
: The thread name of the log message, in square brackets[...]
, such as[main]
%-5level
: The log level of the log message, in uppercase letters, padded with spaces to 5 characters, such asINFO
,DEBUG
,WARN
%logger{36}
: The logger name of the log message, truncated to 36 characters, such ascom.example.App
,org.springframework.boot.web.embedded.tomcat.TomcatWebServer
-
: A dash symbol-
to separate the elements%msg
: The log message itself, which can be any text or object, such asApplication started successfully
%n
: A newline character\n
to end the line
The result of this pattern will look like this:
2024-02-10T11:59:13.123+00:00 [main] INFO com.example.App - Application started successfully
The pattern elements that we can use are defined by the Logback framework, and they can be found in the Logback documentation. We can also use custom or user-defined elements, such as variables, colors, markers, etc., by using the %X
, %highlight
, %marker
, etc. tokens.
The logging format that we specify in the application.yml
file will override the default logging format that is configured by Spring Boot and Logback. However, we can also use the spring.profiles.active
property to activate different logging formats for different profiles or environments. For example, if we want to use different logging formats for development and production profiles, we can use the following properties:
spring.profiles.active: dev
---
spring.profiles: dev
logging.pattern.console: "%d{HH:mm:ss.SSS} [%t] %-5level %logger{36} - %msg%n"
---
spring.profiles: prod
logging.pattern.console: "%d{ISO8601} [%t] %-5level %logger{36} - %msg%n"
The ---
symbol is used to separate different sections or documents in the application.yml
file. The spring.profiles.active
property specifies the active profile for the application, which is dev
in this case. The spring.profiles
property specifies the profile for each section, which can be dev
or prod
in this case. The logging.pattern.console
property specifies the pattern for the console output for each profile, which is HH:mm:ss.SSS
for dev
and ISO8601
for prod
in this case.
To run the application with a specific profile, we can use the following command:
mvn spring-boot:run -Dspring-boot.run.profiles=prod
This will run the application with the prod
profile, which will use the ISO 8601 format for the console output.
Step 5: Configure logging destination in the application.yml file
Another aspect of logging configuration that we can customize is the logging destination, which is the place or medium where the log messages are outputted and stored. The logging destination can affect the performance, availability, and security of the log messages.
To configure the logging destination of the application, we can use the logging.file
or logging.path
properties, which allow us to specify the file or folder for the log output. The syntax of the properties is:
logging.file.name: <file-name>
logging.file.path: <folder-path>
The <file-name>
parameter specifies the name of the file for the log output, which can be any valid file name, such as application.log
, myapp.log
, etc. The <folder-path>
parameter specifies the path of the folder for the log output, which can be any valid folder path, such as /var/log
, /tmp
, etc.
For example, if we want to output the log messages to a file named application.log
in the current working directory, we can use the following property:
logging.file.name: application.log
If we want to output the log messages to a file named myapp.log
in the /var/log
folder, we can use the following properties:
logging.file.name: myapp.log
logging.file.path: /var/log
The logging file or path that we specify in the application.yml
file will override the default logging destination that is configured by Spring Boot and Logback. However, we can also use the spring.profiles.active
property to activate different logging destinations for different profiles or environments. For example, if we want to use different logging destinations for development and production profiles, we can use the following properties:
spring.profiles.active: dev
---
spring.profiles: dev
logging.file.name: application-dev.log
---
spring.profiles: prod
logging.file.name: application-prod.log
logging.file.path: /var/log
The ---
symbol is used to separate different sections or documents in the application.yml
file. The spring.profiles.active
property specifies the active profile for the application, which is dev
in this case. The spring.profiles
property specifies the profile for each section, which can be dev
or prod
in this case. The logging.file.name
and logging.file.path
properties specify the file name and folder path for the log output for each profile, which are application-dev.log
and application-prod.log
in the current working directory and /var/log
folder respectively in this case.
To run the application with a specific profile, we can use the following command:
mvn spring-boot:run -Dspring-boot.run.profiles=prod
This will run the application with the prod
profile, which will output the log messages to the file application-prod.log
in the /var/log
folder.
Step 6: Enable logging rotation and compression in the application.yml file
Another aspect of logging configuration that we can customize is the logging rotation and compression, which are the processes of archiving and compressing the log files after a certain time or size. The logging rotation and compression can help to manage the disk space and performance of the log files, as well as to improve the security and retention of the log messages.
To enable logging rotation and compression of the application, we can use the logging.file.max-size
, logging.file.max-history
, and logging.file.total-size-cap
properties, which allow us to specify the size, history, and cap of the log files. The syntax of the properties is:
logging.file.max-size: <size>
logging.file.max-history: <number>
logging.file.total-size-cap: <size>
The <size>
parameter specifies the maximum size of the log file or the total size of the log files, which can be any valid size unit, such as 10MB
, 100KB
, 1GB
, etc. The <number>
parameter specifies the maximum number of the log files to keep, which can be any positive integer, such as 7
, 30
, 365
, etc.
For example, if we want to rotate the log file every 10 MB, and keep up to 7 log files, we can use the following properties:
logging.file.max-size: 10MB
logging.file.max-history: 7
This will rotate the log file every 10 MB, and keep up to 7 log files, such as application.log
, application.log.1
, application.log.2
, …, application.log.7
. The oldest log file will be deleted when a new log file is created.
If we want to compress the log files after rotation, we can use the .gz
or .zip
extension for the log file name, such as:
logging.file.name: application.log.gz
This will compress the log files after rotation, and keep up to 7 compressed log files, such as application.log.gz
, application.log.1.gz
, application.log.2.gz
, …, application.log.7.gz
. The compressed log files will take less disk space than the uncompressed log files.
If we want to limit the total size of the log files, we can use the logging.file.total-size-cap
property, such as:
logging.file.total-size-cap: 100MB
This will limit the total size of the log files to 100 MB, and delete the oldest log files when the cap is reached.
The logging rotation and compression properties that we specify in the application.yml
file will override the default logging rotation and compression behavior that is configured by Spring Boot and Logback. However, we can also use the spring.profiles.active
property to activate different logging rotation and compression settings for different profiles or environments. For example, if we want to use different logging rotation and compression settings for development and production profiles, we can use the following properties:
spring.profiles.active: dev
---
spring.profiles: dev
logging.file.name: application-dev.log
logging.file.max-size: 10MB
logging.file.max-history: 7
---
spring.profiles: prod
logging.file.name: application-prod.log.gz
logging.file.max-size: 10MB
logging.file.max-history: 30
logging.file.total-size-cap: 1GB
The ---
symbol is used to separate different sections or documents in the application.yml
file. The spring.profiles.active
property specifies the active profile for the application, which is dev
in this case. The spring.profiles
property specifies the profile for each section, which can be dev
or prod
in this case. The logging.file.name
, logging.file.max-size
, logging.file.max-history
, and logging.file.total-size-cap
properties specify the file name, size, history, and cap for the log files for each profile, which are different for dev
and prod
in this case.
To run the application with a specific profile, we can use the following command:
mvn spring-boot:run -Dspring-boot.run.profiles=prod
This will run the application with the prod
profile, which will rotate and compress the log files every 10 MB, and keep up to 30 log files with a total size cap of 1 GB.
Example: Compress logs after one day
To configure Spring Boot to compress the logs after one day, you can use the logging.file.max-history
property in the application.yml
file, and set it to 1
. This will keep only one day of log files, and compress the older ones with the .gz
extension. For example:
logging.file.name: application.log.gz
logging.file.max-history: 1
Example: Compress logs after a month
To configure Spring Boot to compress the logs after one month, you can use the same property, but set it to 30
. This will keep 30 days of log files, and compress the older ones with the .gz
extension. For example:
logging.file.name: application.log.gz
logging.file.max-history: 30
You can also use the logging.file.max-size
property to limit the size of each log file, and the logging.file.total-size-cap
property to limit the total size of all log files.
Step 7: Follow the best practices on storing logs
Storing logs is an important part of log management, as it affects the availability, security, and compliance of the log data. Storing logs requires careful planning and implementation, as it involves various factors such as the location, format, size, and retention of the log files. Here are some of the best practices on storing logs:
- Choose a suitable location for your log files. The location of your log files can have a significant impact on the performance, reliability, and security of your logging system. You should choose a location that is accessible, scalable, and resilient, such as a dedicated disk, a network share, a cloud storage, or a log management service. You should also avoid storing your log files on the same device or server as your application, as it can cause performance degradation, resource contention, and security risks. You should also consider the network bandwidth, latency, and cost of transferring your log files to the desired location.
- Use a standard and structured format for your log files. The format of your log files can affect the readability, usability, and interoperability of your log data. You should use a standard and structured format for your log files, such as JSON, XML, CSV, etc. A standard and structured format can make your log files more consistent, parsable, and searchable, as well as more compatible with various tools and systems. You should also use a common and well-defined naming convention for your log files, such as using the date, time, application name, or log level as part of the file name.
- Optimize the size of your log files. The size of your log files can affect the storage capacity, performance, and cost of your logging system. You should optimize the size of your log files by using appropriate log levels, filtering, sampling, and compression techniques. You should use the lowest log level that meets your logging objectives, and filter out any unnecessary or redundant log messages. You should also sample your log messages to reduce the volume and frequency of the log output, and compress your log files to save disk space and bandwidth. You should also use a suitable unit for the size of your log files, such as KB, MB, GB, etc.
- Establish a log retention policy. A log retention policy is a set of rules that defines how long and how much log data you should keep. A log retention policy can help you balance the trade-off between the value and the cost of your log data, as well as comply with any legal or regulatory requirements. You should establish a log retention policy that is aligned with your logging objectives, business needs, and compliance obligations. You should also consider the storage capacity, performance, and cost of your logging system, as well as the relevance, usefulness, and sensitivity of your log data. You should also review and update your log retention policy regularly, and enforce it consistently.
Step 8: Enable automatic log deletion in Spring Boot
One of the ways to implement a log retention policy in Spring Boot is to enable automatic log deletion, which is the process of removing old or obsolete log files after a certain time or size. Automatic log deletion can help you manage the disk space and performance of your log files, as well as improve the security and compliance of your log data.
To enable automatic log deletion in Spring Boot, you can use the logging.file.max-history
and logging.file.total-size-cap
properties, which allow you to specify the history and cap of the log files. The syntax of the properties is:
logging.file.max-history: <number>
logging.file.total-size-cap: <size>
The <number>
parameter specifies the maximum number of the log files to keep, which can be any positive integer, such as 7
, 30
, 365
, etc. The <size>
parameter specifies the maximum total size of the log files to keep, which can be any valid size unit, such as 10MB
, 100KB
, 1GB
, etc.
For example, if we want to delete the log files that are older than 30 days, we can use the following property:
logging.file.max-history: 30
This will delete the log files that are older than 30 days, and keep up to 30 log files.
If we want to delete the log files that exceed 1 GB in total size, we can use the following property:
logging.file.total-size-cap: 1GB
This will delete the oldest log files when the total size of the log files exceeds 1 GB, and keep the log files within the 1 GB cap.
The automatic log deletion properties that we specify in the application.yml
file will override the default log deletion behavior that is configured by Spring Boot and Logback. However, we can also use the spring.profiles.active
property to activate different log deletion settings for different profiles or environments. For example, if we want to use different log deletion settings for development and production profiles, we can use the following properties:
spring.profiles.active: dev
---
spring.profiles: dev
logging.file.max-history: 7
logging.file.total-size-cap: 100MB
---
spring.profiles: prod
logging.file.max-history: 30
logging.file.total-size-cap: 1GB
The ---
symbol is used to separate different sections or documents in the application.yml
file. The spring.profiles.active
property specifies the active profile for the application, which is dev
in this case. The spring.profiles
property specifies the profile for each section, which can be dev
or prod
in this case. The logging.file.max-history
and logging.file.total-size-cap
properties specify the history and cap for the log files for each profile, which are different for dev
and prod
in this case.
To run the application with a specific profile, we can use the following command:
mvn spring-boot:run -Dspring-boot.run.profiles=prod
This will run the application with the prod
profile, which will delete the log files that are older than 30 days or exceed 1 GB in total size.
I want to store logs for a longer duration
If you want to delete logs after let's say, 6 months, you can use the logging.file.max-history
property in the application.yml
file, and set it to 180
. This will keep 180 days of log files, and delete the older ones. For example:
logging.file.name: application.log.gz
logging.file.max-history: 180
Conclusion
In this article, we have discussed some of the best practices and guidelines for logging in Spring Boot applications, such as using a logging framework, using appropriate log levels, using descriptive and consistent messages, using structured and machine-readable formats, and using appropriate appenders and destinations.
We have also provided a comprehensive tutorial on how to use the logging framework Logback with Spring Boot, and how to customize and configure various aspects of logging, such as the log level, the log pattern, the log destination, etc. We have also shown how to use the application.yml
file to control the logging configuration for different profiles or environments.
Logging is a vital part of any software development process, and it can help developers to improve the quality, reliability, and security of their applications. Logging can also help users and operators to monitor, understand, and troubleshoot their systems. Logging requires careful planning, design, and implementation to ensure that it is effective, efficient, and consistent. We hope that this article has provided some useful information and tips on how to log better in Spring Boot.
With all the logging in place, you might consider setting up a monitoring and alerting solution, as the one demonstrated below: