The unexpected results of a missing comma in JavaScript
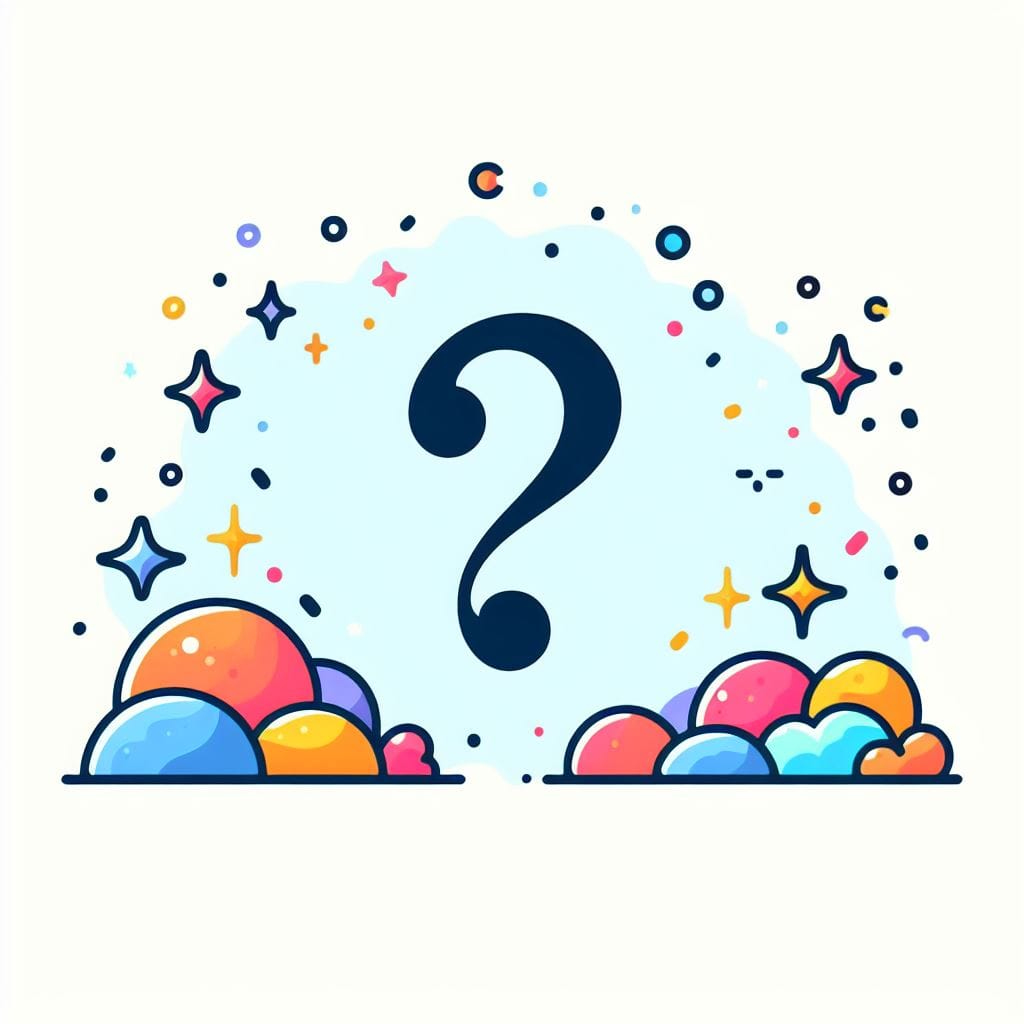
JavaScript is a language full of surprises. Sometimes, a seemingly harmless piece of code can produce unexpected results or even throw errors. After all, the language was initially created in just 10 days.
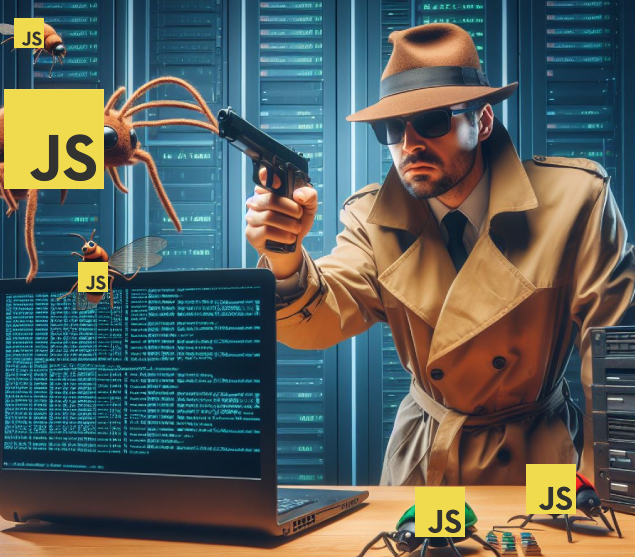
One such example is the missing comma puzzler, which involves an object literal with a missing comma between two properties.
Consider the following code:
var obj = {
a: 1
b: 2
};
What do you think will happen when you run this code? Will it create an object with two properties, a
and b
, or will it throw a syntax error?
The answer is… neither! Instead, it will create an object with a single property, a
, whose value is a labelled statement.
A labelled statement is a way of naming a statement so that it can be referenced by a break
or continue
statement. For example:
loop1: // this is a label
for (let i = 0; i < 10; i++) {
if (i === 5) {
break loop1; // this will break out of the loop with the label loop1
}
console.log(i); // this will print 0, 1, 2, 3, and 4
}
In the case of this puzzler, the label is b
and the statement is 2
. This means that the object obj
is equivalent to:
var obj = {
a: b: 2
};
This is a valid syntax, but it is not very useful. The label b
has no effect on the value of the property a
, which is still 2
. Moreover, the label b
is not accessible outside of the object literal, so it cannot be used by a break
or continue
statement.
What's the best practice?
The solution to this puzzler is simple: always use commas to separate properties in an object literal. Alternatively, you can use the ES6 syntax of shorthand property names, which does not require commas. For example:
let a = 1;
let b = 2;
let obj = { a, b }; // this is equivalent to { a: a, b: b }
This way, you can avoid the missing comma puzzler and create objects that are clear and consistent. JavaScript may be a language full of surprises, but with some attention to detail, you can avoid some of the most common pitfalls and write code that is robust and reliable.
Check out another similar JavaScript puzzler below!
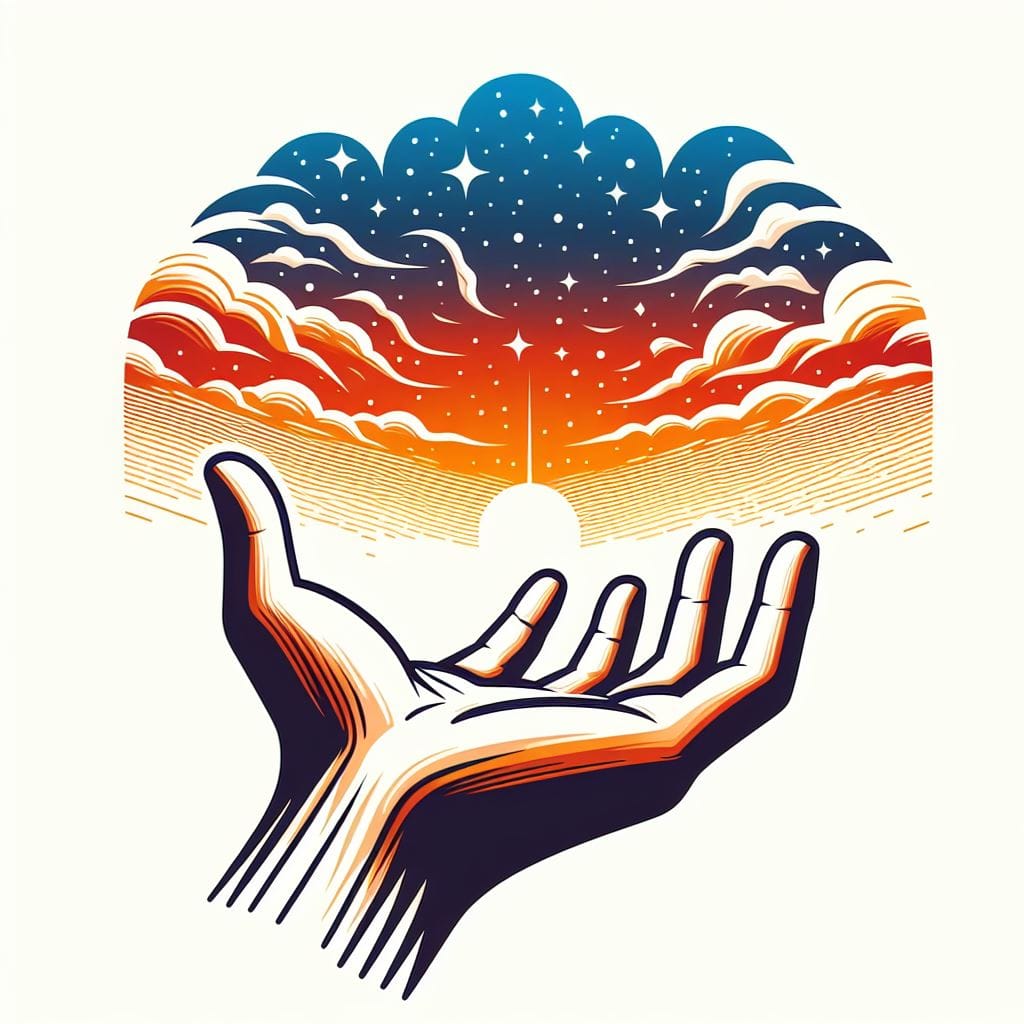