How to Use Regular Expressions in JavaScript
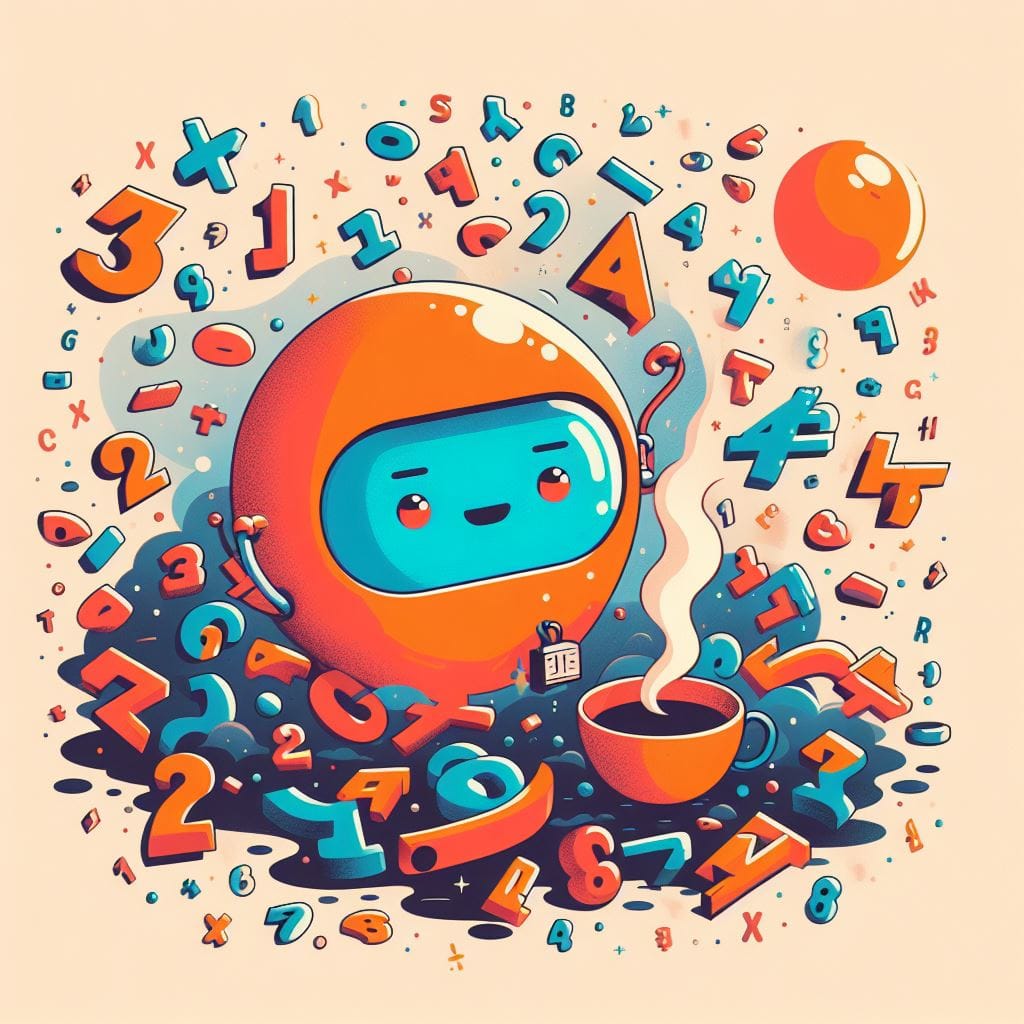
Regular expressions are patterns that can be used to match, search, or replace text in a string. Regular expressions can be very useful for validating user input, extracting data, or manipulating text. In JavaScript, regular expressions are objects that can be created using the RegExp constructor or the literal notation.
What is a regular expression?
A regular expression is a sequence of characters that defines a pattern. For example, the regular expression /[a-z]+/
matches one or more lowercase letters in a string. A regular expression can contain special characters, called metacharacters, that have special meanings. For example, the metacharacter ^
matches the beginning of a string, and the metacharacter $
matches the end of a string. A regular expression can also contain modifiers, called flags, that change the behavior of the pattern. For example, the flag i
makes the pattern case-insensitive, and the flag g
makes the pattern global, meaning it will match all occurrences in the string.
How to create a regular expression?
There are two ways to create a regular expression in JavaScript: using the RegExp constructor or the literal notation. The RegExp constructor takes a string as the first argument, and an optional string of flags as the second argument. For example, the following code creates a regular expression that matches the word “hello” in any case:
let regex = new RegExp("hello", "i");
The literal notation uses two forward slashes (/
) to enclose the pattern, and an optional string of flags after the second slash. For example, the following code creates the same regular expression as above:
let regex = /hello/i;
The literal notation is preferred over the constructor, as it is more concise and avoids the need to escape backslashes (\
) in the pattern.
How to use a regular expression?
Once a regular expression is created, it can be used to perform various operations on a string, such as testing, matching, searching, or replacing. Some of the methods that can be used with regular expressions are:
test()
: This method takes a string as an argument and returns a boolean value indicating whether the string matches the pattern or not. For example, the following code tests if the string “Hello, world!” contains the word “hello” in any case:
let regex = /hello/i;
let str = "Hello, world!";
let result = regex.test(str); // true
match()
: This method takes a regular expression as an argument and returns an array of the matches found in the string, or null if no matches are found. For example, the following code matches all the words that start with “h” in the string “Hello, how are you?”:
let regex = /\bh\w+/g;
let str = "Hello, how are you?";
let result = str.match(regex); // ["Hello", "how"]
search()
: This method takes a regular expression as an argument and returns the index of the first match found in the string, or -1 if no match is found. For example, the following code searches for the word “are” in the string “Hello, how are you?”:
let regex = /are/;
let str = "Hello, how are you?";
let result = str.search(regex); // 10
replace()
: This method takes a regular expression and a replacement string as arguments and returns a new string with the matches replaced by the replacement string. For example, the following code replaces all the occurrences of “o” with “0” in the string “Hello, world!”:
let regex = /o/g;
let str = "Hello, world!";
let result = str.replace(regex, "0"); // "Hell0, w0rld!"
Conclusion
Regular expressions are powerful tools that can be used to manipulate text in JavaScript.
However, regular expressions can also be complex and hard to read, so it is important to use them wisely and carefully, and follow some general guidelines to write a good regular expression.