How to Use Recursion in Python
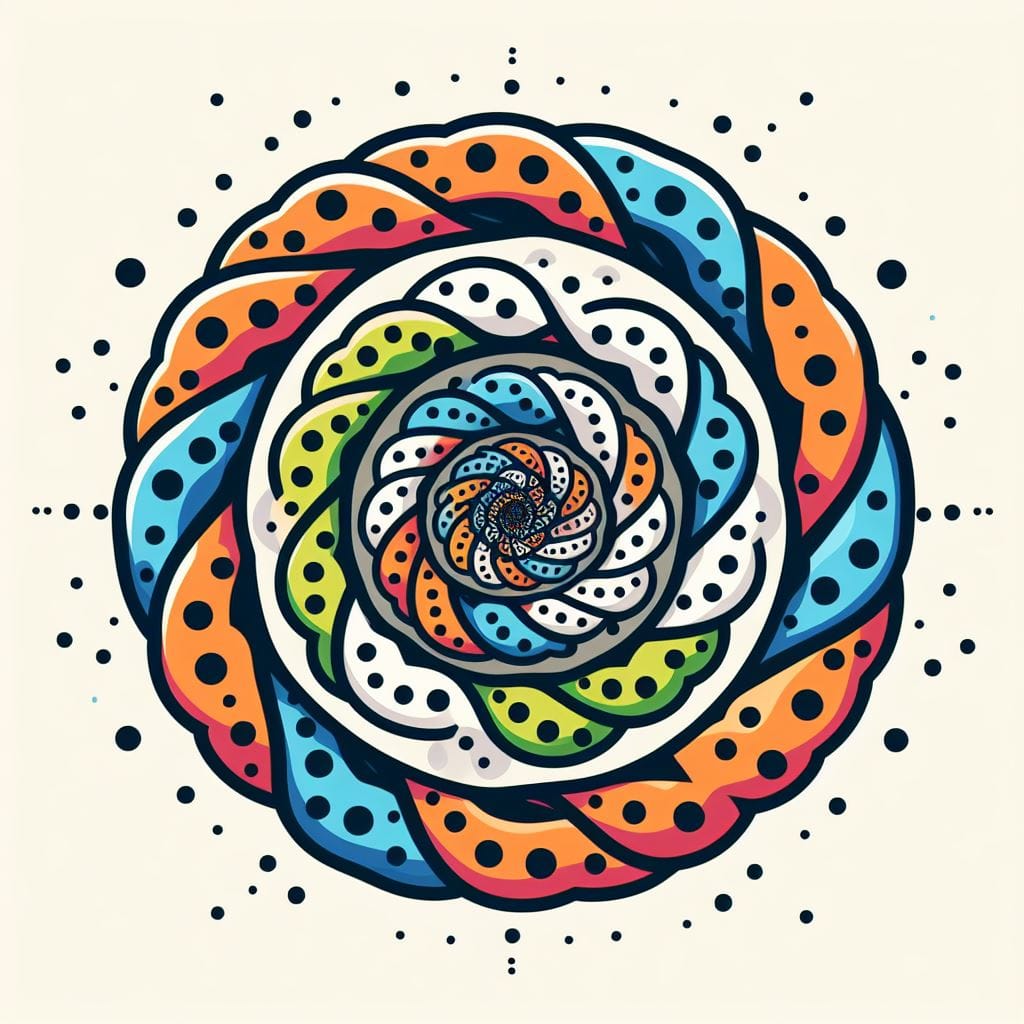
Recursion is a technique in programming where a function calls itself repeatedly until a base case is reached. Recursion can be used to solve problems that have a recursive structure, such as factorial, Fibonacci, tree traversal, etc.
What is a recursive function?
A recursive function is a function that calls itself within its own body. For example, the following function is a recursive function that calculates the factorial of a positive integer n:
def factorial(n):
# base case: 0! = 1
if n == 0:
return 1
# recursive case: n! = n * (n-1)!
else:
return n * factorial(n-1)
Python recusrion code (factorials)
The function factorial has two cases: a base case and a recursive case. The base case is the simplest case that can be solved directly, without any further recursion. The recursive case is the general case that can be reduced to a simpler case by calling the function itself with a smaller argument.
How does recursion work?
When a recursive function is called, the function creates a new stack frame on the call stack, which stores the local variables and parameters of the function. The function then checks if the base case is satisfied. If yes, the function returns the result and pops the stack frame. If no, the function makes a recursive call with a smaller argument, which creates another stack frame on the call stack. This process repeats until the base case is reached, and then the results are returned from the bottom to the top of the call stack.
For example, when we call factorial(3)
, the following steps happen:
- The function creates a stack frame for factorial(3) and checks if n == 0. Since it is not, the function returns 3 * factorial(2).
- The function creates a stack frame for factorial(2) and checks if n == 0. Since it is not, the function returns 2 * factorial(1).
- The function creates a stack frame for factorial(1) and checks if n == 0. Since it is not, the function returns 1 * factorial(0).
- The function creates a stack frame for factorial(0) and checks if n == 0. Since it is, the function returns 1 and pops the stack frame.
- The function returns 1 * 1 = 1 to the previous stack frame and pops the stack frame.
- The function returns 2 * 1 = 2 to the previous stack frame and pops the stack frame.
- The function returns 3 * 2 = 6 to the previous stack frame and pops the stack frame.
- The final result is 6.
What are the advantages and disadvantages of recursion?
Recursion has some advantages and disadvantages compared to iterative solutions. Some of the advantages are:
- Recursion can make the code more concise and elegant, as it can express the problem in terms of itself.
- Recursion can handle complex problems that have a recursive structure, such as tree traversal, backtracking, dynamic programming, etc.
Some of the disadvantages are:
- Recursion can cause a lot of overhead, as it creates multiple stack frames on the call stack, which consumes memory and time.
- Recursion can cause a stack overflow error, if the recursion depth exceeds the limit of the call stack.
- Recursion can be hard to debug and understand, as it involves multiple levels of execution and return.
How to write a good recursive function?
To write a good recursive function, we need to follow some general guidelines:
- Identify the base case and the recursive case of the problem, and make sure they are mutually exclusive and exhaustive.
- Make sure the recursive case reduces the problem to a simpler subproblem, and the recursion eventually reaches the base case.
- Avoid unnecessary or duplicate calculations, and use memoization or dynamic programming if needed.
- Test the function with small and large inputs, and check for edge cases and errors.
Conclusion
Recursion is a powerful technique in programming that can solve problems that have a recursive structure. However, recursion also has some drawbacks, such as memory and time overhead, stack overflow, and difficulty in debugging. Therefore, we need to use recursion wisely and carefully, and follow some general guidelines to write a good recursive function. I hope this article was helpful and interesting to read. 😊