How to Use Classes and Objects in C++
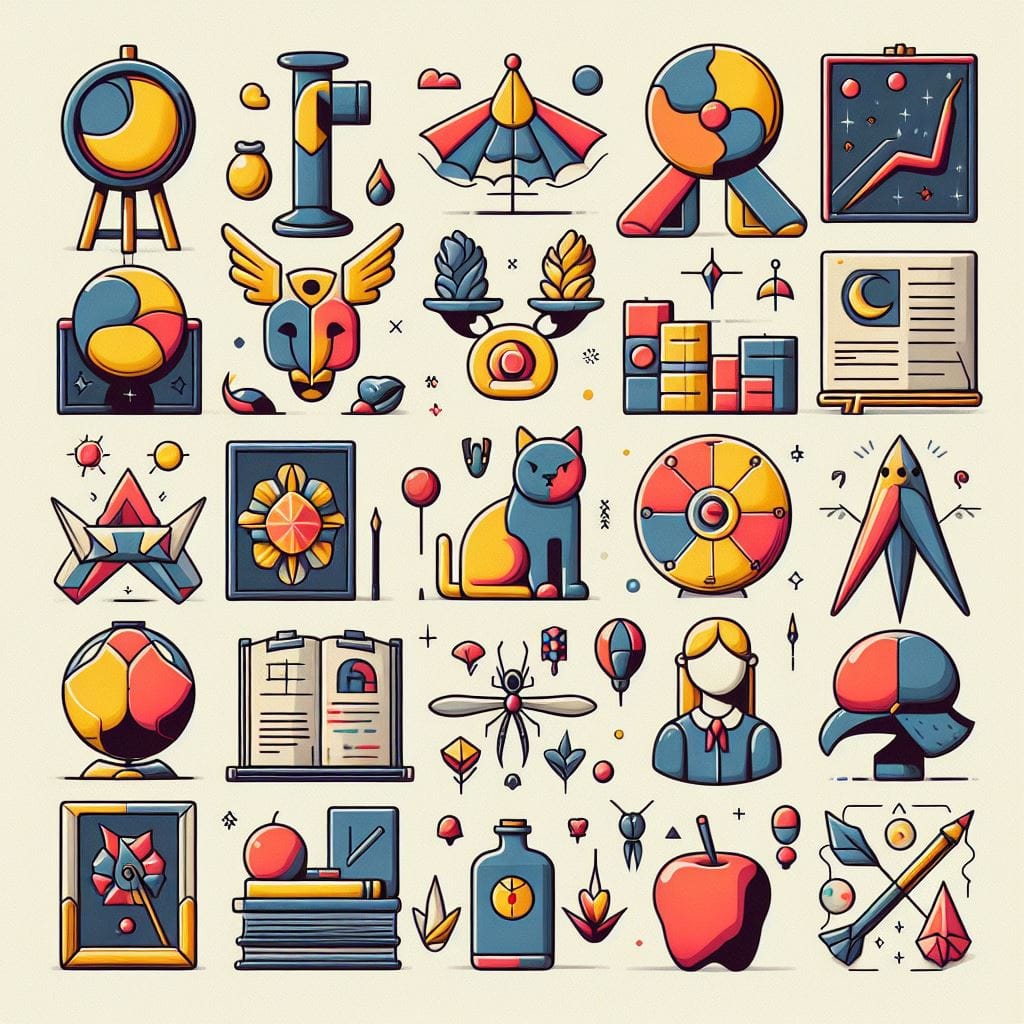
Classes and objects are fundamental concepts in object-oriented programming (OOP), which is a paradigm that organizes data and behavior into reusable and modular units. Classes and objects can be very useful for modeling real-world entities, such as cars, animals, or users. In C++, classes and objects can be created using the class keyword and the constructor syntax.
What is a class?
A class is a blueprint that defines the attributes and methods of a type of object. For example, the following class defines a type of object called Car, which has attributes such as color, model, and speed, and methods such as start, stop, and accelerate:
class Car {
// private attributes
private:
string color;
string model;
int speed;
// public methods
public:
// constructor
Car(string c, string m) {
color = c;
model = m;
speed = 0;
}
// start the car
void start() {
cout << "The car is started." << endl;
}
// stop the car
void stop() {
cout << "The car is stopped." << endl;
}
// accelerate the car
void accelerate(int a) {
speed += a;
cout << "The car is accelerated by " << a << " km/h." << endl;
}
// get the color of the car
string getColor() {
return color;
}
// get the model of the car
string getModel() {
return model;
}
// get the speed of the car
int getSpeed() {
return speed;
}
};
The class has two sections: private and public. The private section contains the attributes that are only accessible within the class, and the public section contains the methods that are accessible outside the class. The constructor is a special method that is invoked when an object of the class is created, and it is used to initialize the attributes of the object.
What is an object?
An object is an instance of a class, which means it has the attributes and methods defined by the class. For example, the following code creates two objects of the class Car, and calls some of their methods:
// create an object of Car with color red and model BMW
Car car1("red", "BMW");
// create another object of Car with color blue and model Toyota
Car car2("blue", "Toyota");
// call the start method of car1
car1.start();
// call the accelerate method of car1 with argument 10
car1.accelerate(10);
// call the stop method of car1
car1.stop();
// call the getColor method of car2 and print the result
cout << "The color of car2 is " << car2.getColor() << endl;
// call the getModel method of car2 and print the result
cout << "The model of car2 is " << car2.getModel() << endl;
// call the getSpeed method of car2 and print the result
cout << "The speed of car2 is " << car2.getSpeed() << " km/h." << endl;
The output of the code is:
The car is started.
The car is accelerated by 10 km/h.
The car is stopped.
The color of car2 is blue
The model of car2 is Toyota
The speed of car2 is 0 km/h.
An object can be created using the constructor syntax, which takes the arguments for the attributes of the object. An object can access the methods of the class using the dot operator (.
).
Conclusion
Classes and objects are essential concepts in object-oriented programming, which can help to create modular and reusable code.
Classes are blueprints that define the attributes and methods of a type of object, and objects are instances of classes that have the attributes and methods of the class. Classes and objects can be created and used in C++ using the class keyword and the constructor syntax.