How to Use Arrays in Java
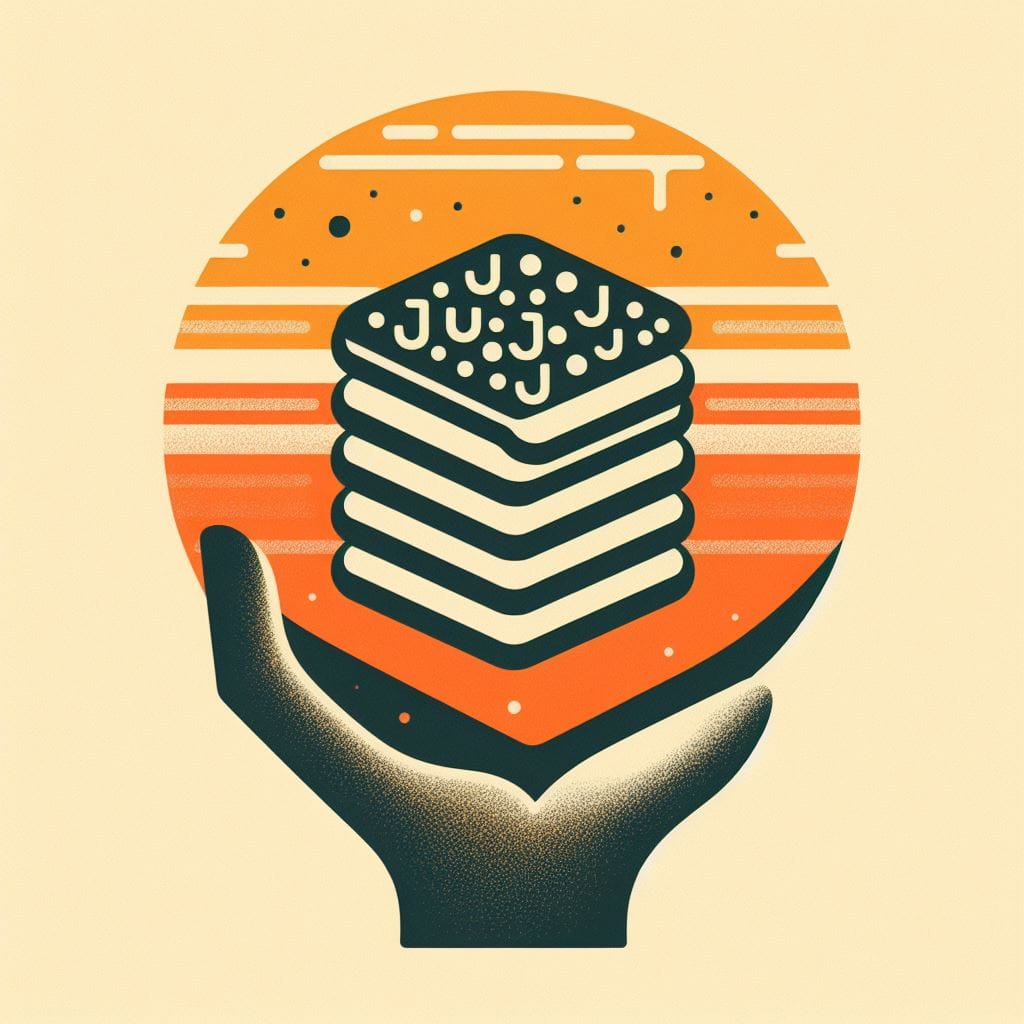
Arrays are data structures that can store multiple values of the same type in a contiguous memory location. Arrays can be very useful for storing and manipulating data, such as numbers, strings, or objects. In Java, arrays are objects that can be created using the new operator or the literal notation.
What is an array?
An array is a collection of elements of the same type, arranged in a fixed-size sequence. For example, the following array can store 5 integers:
int[] arr = new int[5]; // create an array of size 5
The elements of an array can be accessed by their index, which is a zero-based integer that represents their position in the array. For example, the following code assigns and prints the values of the array elements:
arr[0] = 10; // assign the first element
arr[1] = 20; // assign the second element
arr[2] = 30; // assign the third element
arr[3] = 40; // assign the fourth element
arr[4] = 50; // assign the fifth element
System.out.println(arr[0]); // print the first element
System.out.println(arr[1]); // print the second element
System.out.println(arr[2]); // print the third element
System.out.println(arr[3]); // print the fourth element
System.out.println(arr[4]); // print the fifth element
The output of the code is:
10
20
30
40
50
An array can also be created using the literal notation, which is a shorthand way of specifying the values of the array elements. For example, the following code creates an array of 3 strings:
String[] arr = {"Hello", "World", "!"}; // create an array with 3 elements
The length of an array can be obtained using the length
property, which returns the number of elements in the array. For example, the following code prints the length of the array:
System.out.println(arr.length); // print the length of the array
The output of the code is:
3
How to use an array?
Once an array is created, it can be used to perform various operations on the data, such as sorting, searching, copying, or iterating. Some of the methods that can be used with arrays are:
Arrays.sort()
: This method takes an array as an argument and sorts the elements in ascending order. For example, the following code sorts an array of integers:
int[] arr = {5, 3, 1, 4, 2}; // create an unsorted array
Arrays.sort(arr); // sort the array
System.out.println(Arrays.toString(arr)); // print the sorted array
The output of the code is:
[1, 2, 3, 4, 5]
Arrays.binarySearch()
: This method takes an array and a key as arguments and returns the index of the key in the array, or -1 if the key is not found. The array must be sorted before using this method. For example, the following code searches for the key 4 in the array:
int[] arr = {1, 2, 3, 4, 5}; // create a sorted array
int key = 4; // define the key to search
int index = Arrays.binarySearch(arr, key); // search for the key in the array
System.out.println(index); // print the index of the key
The output of the code is:
3
Arrays.copyOf()
: This method takes an array and a new length as arguments and returns a copy of the array with the new length. If the new length is larger than the original length, the extra elements are filled with the default values of the array type. For example, the following code copies an array of 3 elements to an array of 5 elements:
Java
int[] arr = {1, 2, 3}; // create an array of 3 elements
int[] copy = Arrays.copyOf(arr, 5); // copy the array to an array of 5 elements
System.out.println(Arrays.toString(copy)); // print the copied array
AI-generated code. Review and use carefully. More info on FAQ.
The output of the code is:
[1, 2, 3, 0, 0]
Arrays.equals()
: This method takes two arrays as arguments and returns a boolean value indicating whether the two arrays are equal or not. Two arrays are equal if they have the same length and the same elements in the same order. For example, the following code compares two arrays:
int[] arr1 = {1, 2, 3}; // create an array of 3 elements
int[] arr2 = {1, 2, 3}; // create another array of 3 elements
boolean result = Arrays.equals(arr1, arr2); // compare the two arrays
System.out.println(result); // print the result
The output of the code is:
true
How to use ArrayList and LinkedList in Java?
ArrayList and LinkedList are two classes that implement the List interface, which is a subinterface of the Collection interface. A List is an ordered collection of elements that allows duplicates and null values. A List can be used as a dynamic array that can grow and shrink as needed.
ArrayList and LinkedList have some similarities and differences, which are summarized in the following table:
ArrayList | LinkedList |
---|---|
This class uses a dynamic array to store the elements in it. | This class uses a doubly linked list to store the elements in it. |
Manipulation with ArrayList is slow because it internally uses an array. If any element is removed from the array, all the other elements are shifted in memory. | Manipulation with LinkedList is faster than ArrayList because it uses a doubly linked list, so no bit shifting is required in memory. The list is traversed and the reference link is changed. |
An ArrayList class can act as a list only because it implements List only. | LinkedList class can act as a list and queue both because it implements List and Deque interfaces. |
ArrayList is better for storing and accessing data. | LinkedList is better for manipulating data. |
The memory location for the elements of an ArrayList is contiguous. | The location for the elements of a linked list is not contagious. |
Generally, when an ArrayList is initialized, a default capacity of 10 is assigned to the ArrayList. | There is no case of default capacity in a LinkedList. In LinkedList, an empty list is created when a LinkedList is initialized. |
The following is an example to demonstrate the usage of ArrayList and LinkedList in Java:
import java.util.*;
class TestArrayLinked {
public static void main (String args []) {
// create an ArrayList of integers
List<Integer> al = new ArrayList<Integer> ();
// add some elements to the ArrayList
al.add (10);
al.add (20);
al.add (30);
al.add (40);
al.add (50);
// print the ArrayList
System.out.println ("ArrayList: " + al);
// create a LinkedList of strings
List<String> ll = new LinkedList<String> ();
// add some elements to the LinkedList
ll.add ("Hello");
ll.add ("World");
ll.add ("!");
// print the LinkedList
System.out.println ("LinkedList: " + ll);
// remove the first element from the ArrayList
al.remove (0);
// print the updated ArrayList
System.out.println ("ArrayList after removal: " + al);
// remove the last element from the LinkedList
ll.remove (ll.size () - 1);
// print the updated LinkedList
System.out.println ("LinkedList after removal: " + ll);
}
}
The output of the code is:
ArrayList: [10, 20, 30, 40, 50]
LinkedList: [Hello, World, !]
ArrayList after removal: [20, 30, 40, 50]
LinkedList after removal: [Hello, World]
Conclusion
Arrays are basic data structures that can store multiple values of the same type in a fixed-size sequence.
Arrays can be created using the new operator or the literal notation, and can be accessed by their index. Arrays can be used to perform various operations on the data, such as sorting, searching, copying, or iterating. Arrays are very useful for storing and manipulating data in Java.