A practical guide to using the JSONB type in PostgreSQL
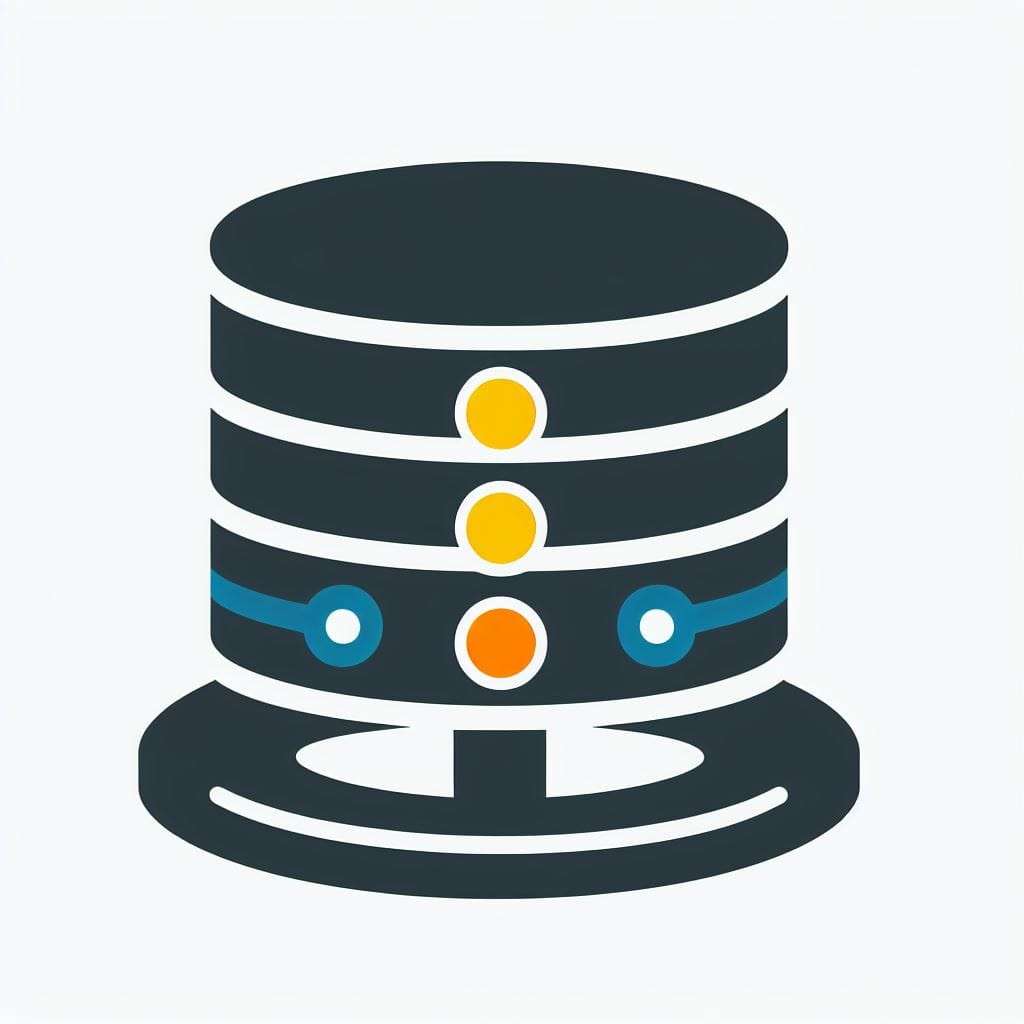
JSONB, or JSON Binary, is a more efficient way to store and manipulate JSON data in PostgreSQL. It stores data in a decomposed binary format, which allows for faster access to individual elements within the JSON document. It is the recommended way to store JSON data in PostgreSQL.
Why Use JSONB in PostgreSQL?
- Indexing: JSONB supports GIN (Generalized Inverted Index) indexing, which can significantly speed up query performance.
- Query Performance: JSONB provides better performance for complex queries involving JSON data.
- Data Manipulation: JSONB allows for more advanced data manipulation capabilities, such as deleting keys from the JSON document.
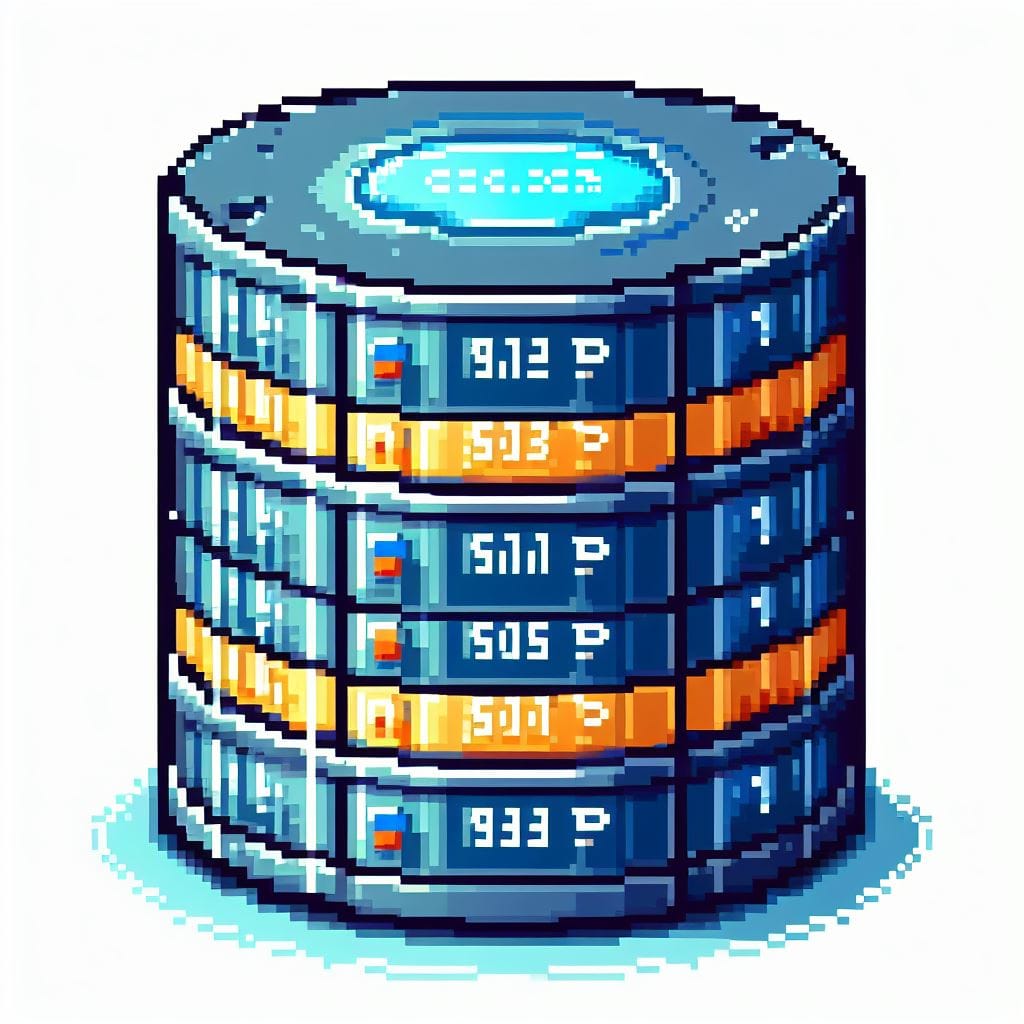
JSONB Operations in PostgreSQL
Working with JSONB in PostgreSQL involves a set of operations that allow you to create, query, update, and manipulate JSONB data efficiently.
Creating JSONB Data
To store data in a JSONB format, you first need to create a table with a JSONB column. Here’s how you can create a table for storing user profiles:
CREATE TABLE user_profiles (
id SERIAL PRIMARY KEY,
profile JSONB NOT NULL
);
Inserting JSONB Data
Once you have a table with a JSONB column, you can insert data into it. Here’s an example of inserting a user profile with various attributes:
INSERT INTO user_profiles (profile)
VALUES ('{"name": "John Doe", "age": 30, "interests": ["coding", "chess"]}');
Selecting JSONB Data
Selecting data from a JSONB column is similar to selecting from any other data type. However, you have the added benefit of using JSONB operators and functions to query specific elements within the JSONB document. Here’s how you can select profiles of users who are interested in chess:
SELECT *
FROM user_profiles
WHERE profile @> '{"interests": ["chess"]}';
Updating JSONB Data
Updating JSONB data can be done using the jsonb_set
function to change existing values, or the ||
operator to add new key-value pairs. Here’s how you can update a user’s age and add a new interest:
UPDATE user_profiles
SET profile = jsonb_set(profile, '{age}', '31') || '{"interests": ["puzzles"]}'
WHERE profile->>'name' = 'John Doe';
Deleting from JSONB Data
To remove a key and its value from a JSONB document, you can use the -
operator. Here’s how to remove the ‘interests’ key from a user’s profile:
UPDATE user_profiles
SET profile = profile - 'interests'
WHERE profile->>'name' = 'John Doe';
Indexing JSONB Data
For performance optimization, especially with large datasets, you can create indexes on JSONB columns. Here’s how to create a GIN index on the profile
column:
CREATE INDEX idxgin ON user_profiles USING GIN (profile);
Real-Life Example: Inventory Management System
An inventory management system needs to track a wide range of product details, which may change frequently. Using JSONB, you can store this dynamic information efficiently.
Schema Design with JSONB:
CREATE TABLE inventory (
id SERIAL PRIMARY KEY,
product_name VARCHAR(255) NOT NULL,
product_details JSONB NOT NULL
);
Inserting Data with JSONB:
INSERT INTO inventory (product_name, product_details)
VALUES
('Widget', '{"manufacturer": "WidgetCo", "specs": {"weight": "1kg", "color": "blue"}}'),
('Gadget', '{"manufacturer": "GadgetCorp", "specs": {"dimensions": "5x2x8", "power": "battery-operated"}}');
Querying Data with JSONB: To find all widgets in blue color, you can use the following query:
SELECT product_name, product_details
FROM inventory
WHERE product_details @> '{"specs": {"color": "blue"}}';
Strategic Use of JSONB in PostgreSQL
JSONB is a versatile data type in PostgreSQL that is particularly useful when dealing with unstructured or semi-structured data. It shines in scenarios where the schema is not fixed, where data integrity is paramount, and where quick read access is required. Below are some specific use cases where JSONB is the preferred choice, along with additional examples to illustrate its practical applications.
Changelogs
In systems where tracking changes over time is crucial, such as version control or audit logs, JSONB is invaluable. By storing a JSONB dump of the changed row along with a diff from the old state, you can maintain a comprehensive changelog without the overhead of relational schema updates.
Example:
CREATE TABLE event_logs (
thing_id INT,
changed_at TIMESTAMP,
state JSONB,
diff JSONB
);
External API Data
When integrating with external APIs, the data structure may not be under your control and can change without notice. Storing API responses in a JSONB column ensures that all data is captured, regardless of its structure. Using a typed view allows for easy access to structured data while preserving the ability to adapt to changes.
Example:
CREATE VIEW typed_api_data AS
SELECT (data->>'amount')::integer
FROM api_data;
Search Parameters
For applications utilizing search engines like Elasticsearch, storing search parameters in JSONB allows for flexibility in the search criteria and the ability to quickly adapt to new search features or changes in the search engine’s API.
Example:
CREATE TABLE search_queries (
query_id SERIAL PRIMARY KEY,
parameters JSONB
);
Dynamic Configuration
JSONB is ideal for storing configuration parameters that may vary between environments or need to be updated on-the-fly without database migrations.
Example:
CREATE TABLE app_config (
config_name VARCHAR(255) NOT NULL,
config_values JSONB NOT NULL
);
User Preferences
Storing user preferences in JSONB allows for a personalized experience where each user can have a unique set of preferences without the need for a complex relational setup.
Example:
CREATE TABLE user_preferences (
user_id INT REFERENCES users(id),
preferences JSONB
);
To JSONB, or not?
When to Consider Using JSONB
- Dynamic Schema: JSONB can be useful when dealing with a schema that changes extremely frequently.
- Complex Data Structures: For deeply nested and complex data structures, JSONB is an option, but evaluate if this complexity can be reduced or better managed with a relational design.
- Full-Text Search: JSONB supports full-text search within JSON documents, but consider whether a dedicated search engine might better serve your needs.
- Data Integrity: JSONB ensures data integrity for JSON formats, but ask yourself if this data needs to be in JSON format or if it could be normalized.
When to Prefer Traditional Relational Models
- Simple, Flat Data: Traditional relational columns are more efficient for simple and flat data structures. They should be your default choice.
- Heavy Write Operations: The overhead of JSONB can slow down write-heavy applications. In such cases, a relational model is likely to perform better.
- Data Normalization: Relational models excel at avoiding data redundancy and ensuring data integrity through normalization. Use them whenever possible.
Important Considerations When Using JSONB in PostgreSQL
The use of JSONB in PostgreSQL should be approached with caution and a clear understanding of its implications. While JSONB offers flexibility for storing JSON data, it is not always the optimal choice for every situation.
Data Manipulation: Be aware that any modification to a JSONB value, no matter how small, necessitates rewriting the entire value to disk. This can lead to inefficiencies, particularly with large JSON documents.
Complexity: Frequent manipulation of complex structures or arrays within JSONB can become cumbersome and may result in less efficient operations compared to a normalized relational model.
Data Integrity: JSONB relinquishes the database’s ability to enforce data type checks. This means there is a risk of storing incorrect data types, such as entering a string where a date is expected.
Performance: While JSONB supports indexing which can aid in query performance, it is crucial to design your queries carefully. Inefficient queries can negate the benefits of indexing and lead to performance issues.
Use Case Appropriateness: JSONB is best used in scenarios where the schema is dynamic and subject to frequent changes. However, if your data is simple, flat, and does not require the flexibility offered by JSONB, traditional relational columns may be more suitable.
Normalization vs. Flexibility: A properly normalized model is often preferable for maintaining data integrity and avoiding redundancy. JSONB should be considered when normalization is not feasible or would lead to performance degradation.
Conclusion
JSONB in PostgreSQL is a powerful tool for developers dealing with dynamic and complex data structures.
It combines the flexibility of JSON with the performance benefits of a binary format, making it an excellent choice for modern applications that require efficient data storage and retrieval.
However, it should be employed when its advantages clearly outweigh the limitations. Developers must remain vigilant about the trade-offs, ensuring that the use of JSONB aligns with the application’s performance requirements and data integrity needs. By adopting a pragmatic approach to JSONB, one can harness its strengths while maintaining the robustness and efficiency of the database system.
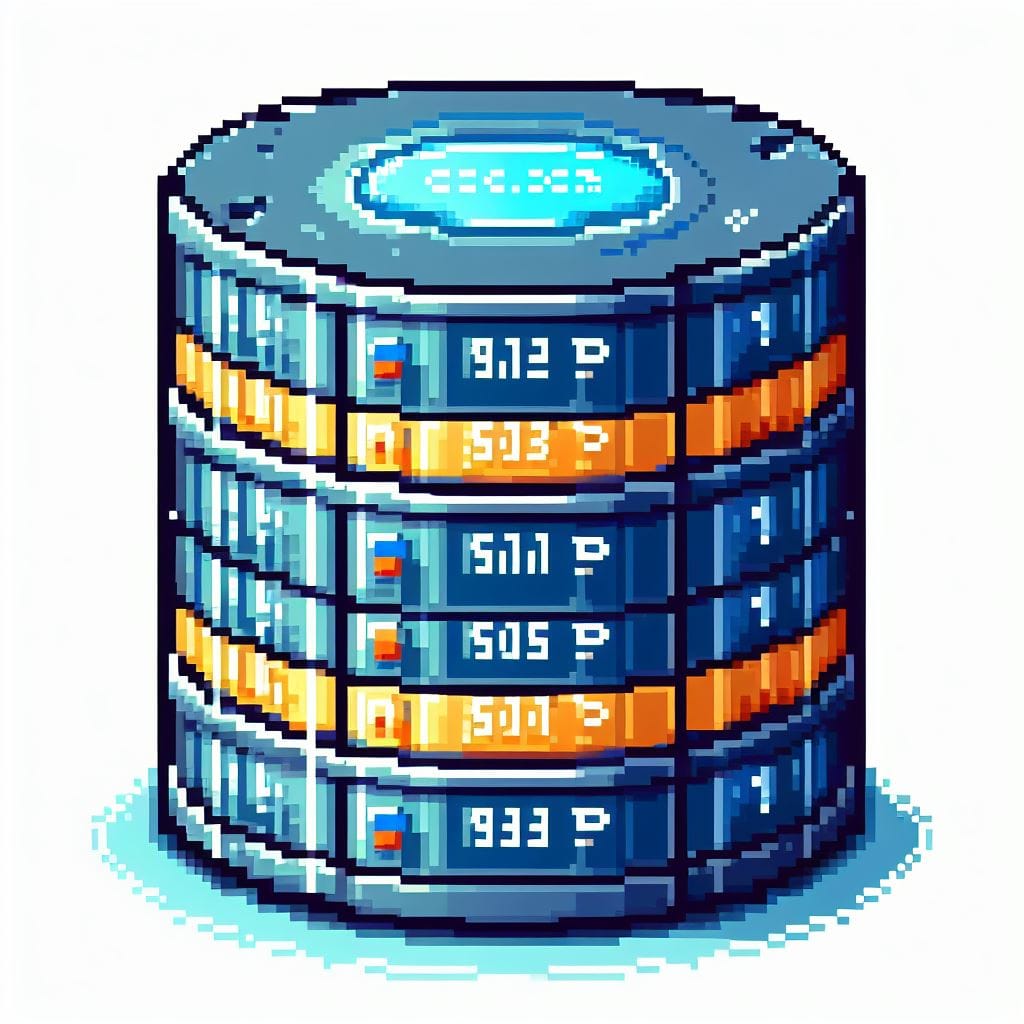